This is the 5th article in a series of articles designed to introduce iOS and Android developers to C#, Silverlight, and Windows Phone 7 application development.
These days pretty much everyone carries a cellular phone and an exponentially increasing number of people are carrying smart phones. Whether we got our smart phone from Apple, Microsoft, or cooked it up in our basement with a roll of duct tape, a bubble gum wrapper, and a spring from a ballpoint pen – chances are our smart phones have some form of location awareness.
These days we can use location awareness in our phones to do everything from the now-ubiquitous turn-by-turn directions to use applications that harness the cloud, social networking, and GPS to find nearby restaurants or speed traps or give us electronic rewards for “checking in” at places.
In this article I’m going to talk specifically about using maps in Windows Phone 7. I’ll start with a quick review of the map capabilities available to iOS developers and then move on to a discussion of including Bing Maps controls in your WP7 application.
Using Maps in iOS
One thing to keep in mind when working with maps in iOS is that the code you write to read latitude and longitude directly from the location sensor is actually different (though sometimes related) from the code you write to work with maps.
To start working with maps in your iOS application, you add the MapKit framework to your product and put an MKMapView control somewhere within a parent view or window. Once you have a map view in place, you can create a class that conforms to the MKMapViewDelegate protocol which lets you supply custom views for map annotations as well as react to various events related to the annotation views on the map. You can change the visible region of the map, control how the map is rendered (e.g. regular map view or satellite view, etc), center the map on a particular location, and even choose whether or not the pulsing blue dot appears on the map to indicate the user’s current position.
Finally, you can add annotations to the map. You’re probably pretty familiar with seeing the thumbtacks drop from the sky and land on specific spots on the map in various applications (the hypothetical application below shows the Headquarters of a company called WINNING):
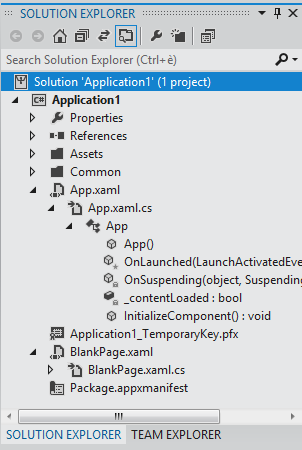
MapKit gives iOS developers a lot of power and lets them integrate all of the power of Google Maps directly into their applications, allowing you to quickly and easily build location-aware applications without having to do any of the heavy lifting. In fact, the iOS SDK has such a robust location system built into it that you can often reverse geocode (get City, State, Zip code, etc., from a latitude/longitude pair) the user’s current location even if they’re using just an iPad over public WiFi.
So the real question here is: can you build location-aware applications with WP7 as easily and quickly as you can with MapKit and iOS? Keep reading to find out.
Using Bing Maps with Windows Phone 7
Given the fact that iOS gets its map drawing capabilities from Google Maps, it should come as no surprise to you that Windows Phone 7 gets its map drawing capabilities from Bing Maps. Just like with iOS, there is a (somewhat fuzzy) gap between the code you write that reads directly from the GPS hardware sensor and the code you write to use the map control. In other words, you can use the map control without ever asking for a current location – the amount of information you glean from the hardware sensors is entirely up to you. There are many applications that make use of maps without ever needing the user’s actual location.
Getting your Bing Maps Developer Account
Probably one of the biggest differences between using MapKit in iOS and using Bing Maps in WP7 is that you need to sign up for an account to use Bing Maps. With iOS, you can start using MapKit as soon as you add the framework to your project and it doesn’t require you to create an account or obtain a license or key.
Bing Maps has varying degrees of licensing levels and usage limits. While it initially has no cost and there are special exemptions for mobile applications, if you’re planning on an enormous number of users utilizing a large number map services, you should have a close look at all of the Bing Maps documentation to make sure you’re not going to end up having to pay for it.
To get your Bing Maps account, go to https://www.bingmapsportal.com/ . From here you can create your new maps account as well as log into an existing account for usage stats, documentation, and various SDK downloads. If you already have a Windows Live ID (you do if you’re an Xbox live user or already a member of the WP7 developer program) then click the Sign In button.
Once you’ve logged in and associated your Live ID with the Bing Maps account you can click the “Create or view keys” link. Here you’ll want to create a new key for your application. In our case, because we’re building Windows Phone 7 applications, we would choose Mobile as the application type. The other application types are beyond the scope of this article but there’s a plethora of information available on them in Microsoft’s documentation as well as on blogs and forums.
The important piece of information that you’re going to need for the next section is the Key. This is a really long blob of letters of varying case, numbers, and simple symbols like dashes or underscores.
Using the Bing Maps Controls
The first thing you’re going to need to do in order to use the map controls in your application is add a reference to the Microsoft.Phone.Controls.Maps assembly.
Once you’ve added a reference to the assembly, you’ll need to make sure that your XAML can utilize that control. To do this, you’ll add a namespace declaration in your page (or whatever the containing control might be) as shown below:
1: <phone:PhoneApplicationPage x:Class="SampleApp.MainPage"
2: xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
3: xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
4: xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
5: xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
6: xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
7: xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
8: xmlns:maps="clr-namespace:Microsoft.Phone.Controls.Maps;assembly=Microsoft.Phone.Controls.Maps"
9: FontFamily="{StaticResource PhoneFontFamilyNormal}"
10: FontSize="{StaticResource PhoneFontSizeNormal}"
11: Foreground="{StaticResource PhoneForegroundBrush}"
12: SupportedOrientations="Portrait"
13: Orientation="Portrait"
14: mc:Ignorable="d"
15: d:DesignWidth="480"
16: d:DesignHeight="768"
17: shell:SystemTray.IsVisible="True"
18: DataContext="{Binding Main, Source={StaticResource Locator}}">
Note that I’ve created a XAML prefix called maps that points to the Microsoft.Phone.Controls.Maps assembly. Just adding a reference to the assembly isn’t enough, we need to let Visual Studio know how to instantiate controls with the maps prefix. The map control is a versatile control but also functions just like any other control in that you can drop it into a XAML control hierarchy wherever you feel appropriate.
Before getting into a sample of how this control works, let’s take a look at some of the properties and methods that provide the map’s core functionality:
- LocationToViewportPoint() – This method takes a GeoCordinate object and returns a Point object. This allows you to determine the exact X and Y coordinate (relative to the upper left corner of the map!) of a specific latitude and longitude. This method is comparable to MKMapView’s convertCoordinate:toPointToView: method in iOS.
- OnHeadingChanged – A useful event to which you can subscribe to be notified when the heading of the map changes.
- OnModeChanged – Another useful event that you can use to react to when the map’s mode changes, e.g. when a map changes from satellite view to pure road view.
- ViewportPointToLocation() – This is the opposite of the LocationToViewportPoint method. For those familiar with the MKMapView class in the iOS SDK, the MKMapView equivalent would be the convertPoint:toCoordinateFromView: method.
- Center – This property is a GeoCoordinate object that corresponds to the visual center point of the map.
- CopyrightVisibility – Determines whether or not the Bing copyright message is visible
- CredentialsProvider – This is the license key information that you obtained when signing up for your Bing maps account.
- Heading – Controls the directional heading of the map.
- IsDownloading – You read the value of this property to determine if the map control is downloading support data such as map tiles and other information.
- LogoVisibility – Controls whether or not the Bing logo is visible on the map.
- Mode – This corresponds to the map mode. This property controls many aspects of the map’s appearance and behavior.
Now that we’re familiar with a couple of the key properties and methods of the map control, let’s see what one looks like in XAML:
1: <maps:Map Grid.Row="0" Height="768"
2: ZoomLevel="{Binding MapZoom, Mode=TwoWay}"
3: Center="{Binding MapCenter, Mode=TwoWay}"
4: CredentialsProvider="(INSERT YOUR OWN)"
5: CopyrightVisibility="Collapsed"
6: LogoVisibility="Collapsed">
7: <maps:Map.Mode>
8: <maps:AerialMode ShouldDisplayLabels="false"/>
9: </maps:Map.Mode>
10:
11: <maps:MapItemsControl ItemsSource="{Binding MapObjects}">
12: <maps:MapItemsControl.ItemTemplate>
13: <DataTemplate>
14: <maps:Pushpin Location="{Binding Position}" Opacity="0.8">
15: <StackPanel>
16: <Image Source="/Resources/Images/002-HomeAlt.png" Height="48" Width="48"/>
17: <TextBlock Text="{Binding Name}"/>
18: <TextBlock Text="{Binding Organization}" Foreground="Yellow"/>
19: </StackPanel>
20: </maps:Pushpin>
21: </DataTemplate>
22: </maps:MapItemsControl.ItemTemplate>
23: </maps:MapItemsControl>
24:
25: </maps:Map>
In the preceding code snippet, we’re setting a few basic properties of the map control and actually data binding some of them such as the ZoomLevel and Center. This will allow us to programmatically change the zoom and center of the map in response to whatever events we choose.
Next I set the map mode to an instance of the AerialMode class (remember, XAML is just an instantiation language so every element you see is an instantiation of an object) and I choose to turn off labels like roads, cities, etc. Next, as the first child of the Map control is a MapItemsControl. This control I’ve bound to a list of map objects and I’ve also defined a data template so that I can control the appearance of each of the map pins that appears for each object.
Each of the objects I am binding to my map has a Name property, an Organization property, and a Position property that is of type GeoCoordinate (remember this type from the article on hardware and device services? It’s the same data type you get back when you listen to the GPS with a GeoCoordinateWatcher). Each item in my data-bound list will render itself as an instance of the Pushpin class (nearly identical in function and purpose to MapKit’s MKPinAnnotationView class).
Without further adieu, here’s a screenshot of what my map view looks like directly in my designer:
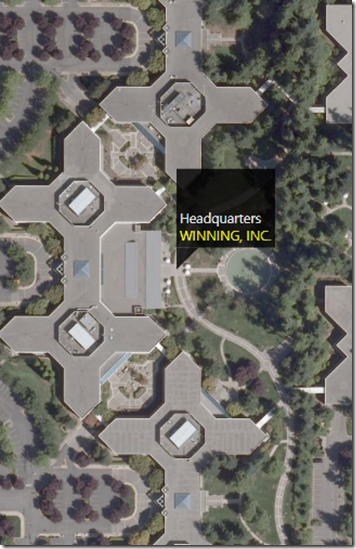
The reason I show you the designer view is because I wanted to point out that the designer is capable of displaying map data directly inside Visual Studio without even using the simulator. This differs from what you see in Xcode on iOS in that Xcode (or Interface Builder if you’re using a version older than Xcode 4) just displays an empty placeholder for the map view.
Here’s the little bit of C# code that creates instances of my MapObject class that corresponds to the preceding screenshot (bonus points if you’ve figured out where the headquarters of WINNING, INC is located in this picture):
1: MapObject mo = new MapObject()
2: {
3: Position = new GeoCoordinate(47.639631, -122.127713),
4: Name = "Headquarters",
5: Organization = "WINNING, INC."
6: };
7: this.MapObjects.Add(mo);
Summary
As I mentioned at the beginning of this article, it is getting harder and harder to find a smart phone that doesn’t have some form of location awareness. iPhones and Windows Phone 7 both have extremely powerful location awareness with GPS receivers capable of determining your current location to within a few yards.
Some applications harness this location awareness by showing you nearby restaurants or houses for sale, others use this awareness to display coupons for nearby stores and yet other applications use these capabilities for gaming or entertainment. The bottom line is that if you are thinking about building an application for Windows Phone 7, ignoring it’s mapping and location capabilities could be a big mistake.
This article showed you how easy it is to work with the map control to display rich, interactive, data-bindable maps filled with whatever content you choose. With map displaying now in your arsenal of WP7 tools, you’re even closer to being able to create virtually any kind of WP7 application for fun or for profit (or hopefully both!)
About the Author
Kevin Hoffman (http://www.kotancode.com/) is a Systems Architect for Oakleaf Waste Management (http://www.oakleafwaste.com/), freelance developer, and author of multiple books including the upcoming WP7 for iPhone Developers and co-author of books such as ASP.NET 4 Unleashed and SharePoint 2007 Development Unleashed. He is the author of the Kotan Code blog and has presented at Apple's WWDC twice and guest lectured at Columbia University on iPhone development.