This article is compatible with the latest version of Silverlight.
Recently I needed to use a web service in order to get some data for my Silverlight application and I found that using an ASMX web service with Silverlight could be quite easy. Though, for people who have never used such service it could be not that easy. So I decided to make this “step-by-step” tutorial and I hope it could be useful to someone.
First let’s create our web service. Create a new project and add an ASMX web service to it. In my example I’ll use the service to get the current date and time, so I name it DateTimeWebService.
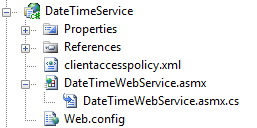
You have probably noticed the clientaccesspolicy.xml file. Basically if there isn’t such file, the Silverlight plug-in can’t access the service. You can find more about this file and the crossdomain.xml file in the FAQ section of this article. Here is how it should look in order to allow any domain to access your web service:
<?xml version="1.0" encoding="utf-8"?>
<access-policy>
<cross-domain-access>
<policy>
<allow-from http-request-headers="*">
<domain uri="*"/>
</allow-from>
<grant-to>
<resource path="/" include-subpaths="true"/>
</grant-to>
</policy>
</cross-domain-access>
</access-policy>
And here are the methods that I define in the asmx.cs file:
[WebMethod]
public string GetDate()
{
return DateTime.Today.ToString( "MMM dd, yyyy" );
}
[WebMethod]
public string GetTime()
{
return DateTime.Now.ToString( "hh:mm:ss" );
}
Now our web service is ready, so let’s create a Silverlight application, which will consume it. Add a Silverlight project to the solution.
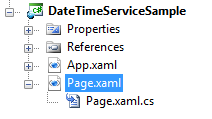
In the XAML we have two TextBlocks and one Button control:
<StackPanel x:Name="LayoutRoot" Background="White">
<TextBlock Width="200" Height="30"></TextBlock>
<TextBlock Width="200" Height="30"></TextBlock>
<Button x:Name="btnUpdateDate" Content="Update Date" Width="100"
Height="30"></Button>
</StackPanel>
We’ll use the TextBlocks to present the date and the time and in the click event of the button we’ll consume the service. But before writing the event we must add a service reference of our service to the Silverlight project:
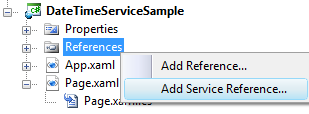
The next step:
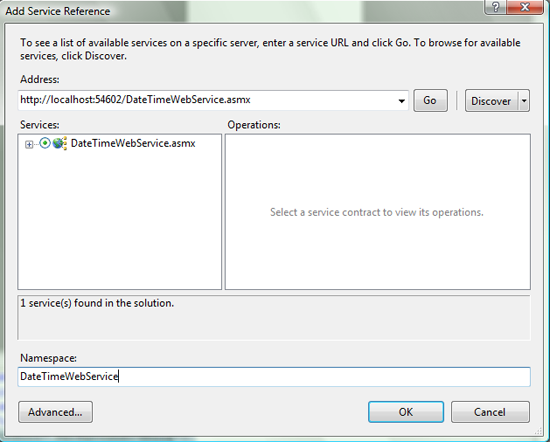
Here you can see the “Go” and the “Discover” buttons. By clicking on the “Discover” button Visual Studio automatically detects the web services that are placed in the current solution. If you want to consume a web service that is placed on a web server just write its address in the address bar.
By clicking “OK” we have a reference to our service added in the Silverlight project. Visual Studio generates all the WSDL (see the FAQ section at the end of the article) you'll need to call the service in several files.
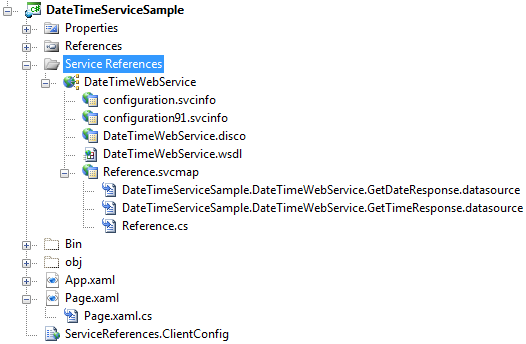
Now let’s create an event handler for the button click event:
private void btnUpdateDate_Click( object sender, RoutedEventArgs e )
{
DateTimeWebService.DateTimeWebServiceSoapClient service =
new DateTimeWebService.DateTimeWebServiceSoapClient();
service.GetDateCompleted +=
new EventHandler<DateTimeWebService.GetDateCompletedEventArgs>(service_GetDateCompleted);
service.GetTimeCompleted +=
new EventHandler<DateTimeWebService.GetTimeCompletedEventArgs>(service_GetTimeCompleted);
service.GetTimeAsync();
service.GetDateAsync();
}
In the service reference there is a SoapClient generated for our DateTimeWebService. Now it is turn to create event handlers for the Completed events of the methods we use. Subsequently, thanks to the event arguments (also generated in the service reference) we can work with the result from our methods, if they return any.
public void service_GetTimeCompleted( object sender,
DateTimeWebService.GetTimeCompletedEventArgs e )
{
Time.Text = string.Format( "The time is {0}", e.Result );
}
private void service_GetDateCompleted( object sender,
DateTimeWebService.GetDateCompletedEventArgs e )
{
Date.Text = string.Format( "Today is {0}.", e.Result );
}
Silverlight works on the client side, so obviously we cannot call the methods synchronous. The Soap client calls our methods asynchronously. And that’s the other reason why we need event handlers for the Completed events.
You see that there is nothing complicated to consume an ASMX service using Silverlight. Here is a live demo of the example with the date. If you are interested you can also find the source code here.
Another example for a Silverlight application consuming an ASMX web service is Silvester ( a Silverlight Twitter widget ), created by Emil Stoychev. You can read the article and take a look at the awesome live demo here.
FAQ
What is a web service?
By the definition of W3C a web service is “a software system designed to support interoperable Machine to Machine interaction over a network”.
What is the client access policy file?
This is a Silverlight Policy file – clientaccesspolicy.xml. Before connecting to a network resource, the Silverlight runtime will try to download this file. It can be used by the sockets class as well.
What is the cross domain file?
This is the Flash policy file – crossdomain.xml. It can be used by the Silverlight runtime like the client access policy file, but it cannot be used along with a sockets class.
Which file is used by the Silverlight runtime?
First the Silverlight runtime tries to download the Silverlight policy file (clientaccesspolicy.xml). If it’s missing, the runtime tries to download the Flash policy file (crossdomain.xml). If none of the files is found, the runtime cannot establish a connection with the network resource.
What is WSDL?
The Web Services Description Language is an XML-based language, which is used for describing web services.
I get error (404) – Not Found, when trying to consume my web service. Why?
When there is a problem with the service, the response will always be 404. So check carefully the code, make sure that the reference, you’ve added, matches the service you want to use and last but not least, don’t forget the clientaccesspolicy.xml and the crossdomain.xml files.
References
http://en.wikipedia.org/wiki/Web_service
http://en.wikipedia.org/wiki/Web_Services_Description_Language
http://msdn.microsoft.com/en-us/library/bb552919.aspx - ASP.NET XML Web Service Basics
http://msdn.microsoft.com/en-us/library/cc645032(VS.95).aspx - Network Security Access Restrictions in Silverlight 2
http://quickstarts.asp.net/QuickStartv20/webservices/default.aspx - ASP.NET Web Services QuickStart Tutorial
http://mtaulty.com/CommunityServer/blogs/mike_taultys_blog/archive/2008/06/30/10548.aspx - Silverlight 2 and ADO.NET Data Services