This article is compatible with the latest version of Silverlight.
Introduction
Only three months after the release of the latest official version of Silverlight 3, a new beta version – Silverlight 4 is already a fact. There are a lot of new things, which deserve to be mentioned, such as Rich Text, drop target, webcam, microphone, etc. Check out the official Silverlight site for more information. However, in this article I decided to show you one very interesting feature, which is taken from WPF, namely it is the implicit styles feature.
What was the situation till now? Whenever you’ve created a style in Silverlight, you were obligated to specify the TargetType as well as an unique Key/Name for the style. You should also explicitly set the Style property of the desired element.
<Style x:Key="ButtonStyle" TargetType="Button">
<Setter Property="Background" Value="Red"/>
<Setter Property="Foreground" Value="Black"/>
<Setter Property="FontSize" Value="16"/>
<Setter Property="Height" Value="55"/>
<Setter Property="Width" Value="140"/>
<Setter Property="Margin" Value="8"/>
<Setter Property="RenderTransformOrigin" Value="0.5,0.5"/>
<Setter Property="RenderTransform">
<Setter.Value>
<RotateTransform Angle="-15"/>
</Setter.Value>
</Setter>
</Style>
.................
<StackPanel>
<Button x:Name="button1" Content="First Button" Style="{StaticResource ButtonStyle}"/>
<Button x:Name="button2" Content="Second Button" Style="{StaticResource ButtonStyle}"/>
</StackPanel>
This is also known as a named style.
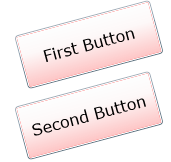
But not any more! Silverlight 4 gives you the ability to create implicit styles.
Creating Implicit Styles
If you omit the Style’s Key and specify only the TargetType, then the Style is automatically applied to all elements of that target type within the same scope (it is implicitly applied). This is typically called a typed style as opposed to a named style, which is the only kind of Style we’ve seen so far.
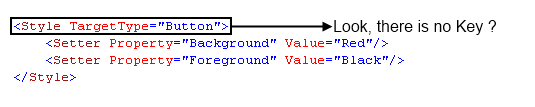
The following example demonstrates you how to create an implicit style, which will be applied to all Buttons in the Application scope.
<Style TargetType="Button">
<Setter Property="Background" Value="Red"/>
<Setter Property="Foreground" Value="Black"/>
<Setter Property="FontSize" Value="16"/>
<Setter Property="Height" Value="55"/>
<Setter Property="Width" Value="140"/>
<Setter Property="Margin" Value="8"/>
<Setter Property="RenderTransformOrigin" Value="0.5,0.5"/>
<Setter Property="RenderTransform">
<Setter.Value>
<RotateTransform Angle="-15"/>
</Setter.Value>
</Setter>
</Style>
.............
<StackPanel>
<Button x:Name="button1" Content="First Button"/>
<Button x:Name="button2" Content="Second Button"/>
</StackPanel>
Note, you shouldn't specify the Button's Style property.
In such an application, all Buttons get this style by default. But each Button can still override its appearance by explicitly setting a different Style or explicitly setting properties.
<Button x:Name="button2" Content="Second Button" Background="Green"/>
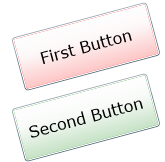
Also, any Button can restore its default Style by setting its Style property to null.
<Button x:Name="button2" Content="Second Button" Style="{x:Null}"/>
The Target Element Must Match Exactly the TargetType
Note, that the TargetType must match exactly for a typed style to be applied. For example, if you specify the Style’s Key, then it’s ok for the target element to be a subclass of the TargetType. But a typed style typically gets applied to elements which type matches exactly! This is done to prevent surprises. For example, you might have created a Style for all ToggleButtons in your application and you don’t want this style to be applied to any CheckBoxes (which derives from the ToggleButton).
Where is the Key for the Keyless Resource?
So far so good, but you may wonder how it is possible a Style to get away with being a member of a ResourceDictionary without having a key. It actually does have a key – it’s just set implicitly. And the implicit key is simply the value of the TargetType (at least this is the situation in WPF, probably it is also true for Silverlight!?!).
Implicit Styles and BasedOn
If you have multiple implicit styles relating to a particular control, then they do not magically combine. For example, if you have the following two styles:
<Style TargetType="Button">
<Setter Property="FontSize" Value="16"/>
</Style>
<Style TargetType="Button">
<Setter Property="Background" Value="Red"/>
<Setter Property="Foreground" Value="Black"/>
</Style>
they won’t get combined. Something more - this will result in a run-time error. However, implicit styles can still use BasedOn. After the refactoring of the previous example it should look like the code below:
<Style x:Key="BasedStyle" TargetType="Button">
<Setter Property="FontSize" Value="16"/>
</Style>
<Style TargetType="Button" BasedOn="{StaticResource BasedStyle}">
<Setter Property="Background" Value="Red"/>
<Setter Property="Foreground" Value="Black"/>
</Style>
Undoubtedly, this is a great addition to the Silverlight styling. You can define implicit styles at any level of your application. Additionally you have the ability to override at any time the implicit style or even to reset it.