This article is compatible with the latest version of Silverlight.
Among the new features introduced with Silverlight 3.0, Out-of-Browser is certainly one that has aroused more interest among developers as it opens very interesting usage scenarios that go beyond the traditional Web application, with version 4.0 this feature has been enhanced with additional options that makes it a viable alternative to classic desktop applications.
Introduction
Out-of-Browser mode allows a Silverlight 3.0 or above application to be detached from its natural host (the browser) and be run by the final user as any desktop application. To enable it all you need to do is select the "Enable application running out of the browser " option available among application properties:

and set available options:
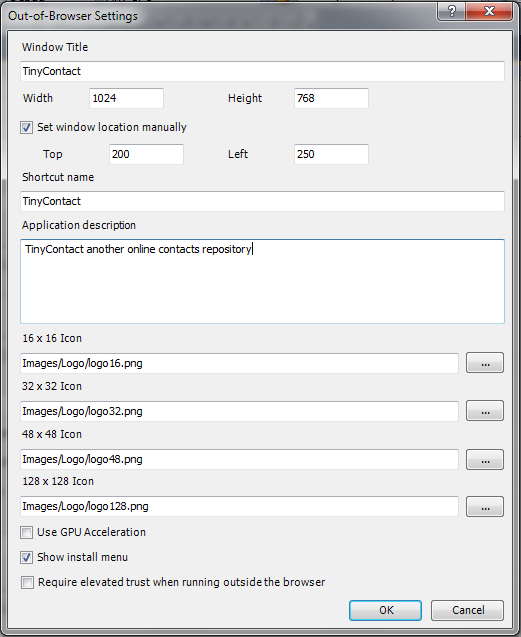
Compared to what’s available in version 3.0 you can immediately observe that you can now set the default window location, remove default installation menu and, especially, enable the "Require Elevated trust when running outside the browser" option through which it's possible to relax the sandbox that hosts the Silverlight application. These settings are then persisted inside AppManifest.xaml file that gets embedded inside application's Xap file.
When "Require Elevated trust when running outside the browser" option is selected when the user choose to install the application locally it is prompted with a ClickOnce like security warning requiring authorization to run application with elevated privileges.
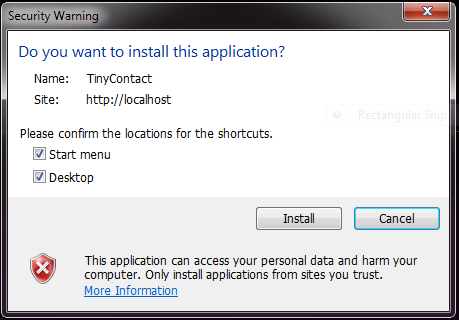
Just after public announce of this feature at PDC 2009, a lot of developers started seeing this feature as the end of the desktop applications but while this opens a lot of opportunities it’s worth mentioning that you can’t still have full control of local machine, for this, a Windows Presentation Foundation 4.0 XBAP application remains the viable alternative.
Detecting when application is running in elevated trust mode
Since some features requires elevated trust and initially your application is run from the browser, detecting whether the application has elevated privileges will become a common scenario inside Silverlight 4.0 applications, that’s why Application class now exposes a HasElevatedPermission property:
bool featureComplete = Application.Current.HasElevatedPermissions;
Once sure about application state we can begin using new features depending on this special mode.
Interacting with application window
Silverlight 4.0 gives you more control of the application hosting window, it’s now possible to change its WindowState, set it TopMost and even change its size at runtime just using Application.MainWindow property as shown below:
Application.Current.MainWindow.Width = 500;
Application.Current.MainWindow.Height = 400;
Application.Current.MainWindow.TopMost = true;
If application is fully trusted you can interact with application window (a.k.a Chrome) without user intervention, this means that you can use this code to easily create a full screen Silverlight application:
public MainPage()
{
InitializeComponent();
if (Application.Current.HasElevatedPermissions && Application.Current.IsRunningOutOfBrowser)
{
Application.Current.MainWindow.WindowState = WindowState.Maximized;
Application.Current.MainWindow.TopMost = true;
}
}
Running the above snippet you’ll see no “Press ESC to exit full screen mode” warning appear, while this give you total control of the ESC key it also means that you’re in charge of closing application window.
When not running in elevated mode interaction with application window it’s available only through a user initiated action (e.g. a Click event)
While not available in current beta it has also been announced that RTM release will include Chrome customization support.
What you can do when application is running with elevated trust
Following is a list of the features available only when application runs inside a relaxed sandbox:
a) Clipboard direct access: When you programmatically access Clipboard class to copy or retrieve text from system clipboard, if application is running with elevated privileges the following dialog does not appear:
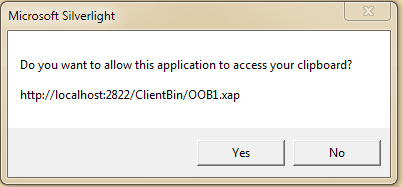
b) Relaxed cross domain restriction: Communicating with services that are not hosted on the application’s site of origin results in Silverlight security model checking for the presence of ClientAccessPolicy.xml or CrossDomain.xml files, if none of those files are detected on target domain, operation returns a security exception.
In full trust mode security check is bypassed and application can safely interact with any domain, as example, following sample will fail in a standard sandboxed application while runs fine on a elevated permission installation:
private void button4_Click(object sender, RoutedEventArgs e)
{
Uri uri = new Uri(@"http://www.ansa.it/web/images/logo_ansa_hp.gif");
WebClient wc = new WebClient();
wc.OpenReadCompleted+=(s,arg)=>
{
if (arg.Error == null)
{
//Save image
}
};
wc.OpenReadAsync(uri);
}
c) Direct access to User folder: Through OpenFileDialog and SaveFileDialog classes any Silverlight application can access local file system, but only inside a full trust application it’s possible access it without user interaction although limited to user folders: My Documents, My Pictures, My Videos , My Music and related subfolders.
Here’s a fragment of code that loads My Pictures folder contents inside a listbox:
if (Application.Current.HasElevatedPermissions)
{
string path = Environment.GetFolderPath(Environment.SpecialFolder.MyPictures);
var files=Directory.EnumerateFiles(path);
ObservableCollection<string> picFiles = new ObservableCollection<string>(files);
listBox1.ItemsSource = picFiles;
}
d) COM Interoperability: Elevated trust mode give you access to COM enabled applications installed on user machine. An example might be a Silverlight 4.0 application that interacts with Office’s Outlook application to popup a send email dialog, following code shows how to do it (a reference to Microsoft.CSharp assembly is required in order to compile the sample)
if (Application.Current.HasElevatedPermissions && ComAutomationFactory.IsAvailable)
{
MessageBox.Show("Ava");
dynamic outlook = ComAutomationFactory.CreateObject("Outlook.Application");
dynamic mail = outlook.CreateItem(0);
mail.To = "john@doe.com";
mail.Subject = "Silverlight 4.0 question";
mail.Body = "I have a question for you";
mail.Display();
}
While this feature is incredibly awesome some caution but be taken in consideration before considering its use:
1) This feature is available on Windows platform only, that’s why code checks ComAutomationFactory.IsAvailable property.
2) Together with trust elevation COM Interoperability might open the door to potential security issues because an application could download an executable to one of the user folders and, via the WScript object, run it, potentially hurting the entire computer, following code shows how simple is to execute a local application in a full trust Silverlight application:
if (Application.Current.HasElevatedPermissions && ComAutomationFactory.IsAvailable)
{
dynamic cmd = ComAutomationFactory.CreateObject("WScript.Shell");
cmd.Run(@"c:\windows\notepad.exe", 1, true);
}
Obviously this works only if user authorized the application but, you know, users can be easily misleaded.
HTML Hosting
Silverlight application running out of browser can now display HTML content using the new WebBrowser control, here’s a code snippet that allows you to navigate to a Uri:
<StackPanel x:Name="LayoutRoot" Background="WhiteSmoke">
<StackPanel Orientation="Horizontal">
<TextBox x:Name="txtUrl" Text="http://www.facebook.com" Width="200" />
<Button Content="Go" Click="OnNavigate" />
</StackPanel>
<WebBrowser x:Name="wb" Width="600" Height="250" />
</StackPanel>
private void OnNavigate(object sender, RoutedEventArgs e)
{
Uri uri = new Uri(txtUrl.Text);
wb.Navigate(uri);
}
In order to display page content application must run in elevated trust mode otherwise WebBrowser control can only safely access pages hosted on application site of origin furthermore being WebBrowser default width and height set to zero a valid size must also be provided.
WebBrowser functionality is available in out-of-browser mode only; running the above sample inside the browser will result in a rectangle rendered as control placeholder.
Notification Window
A new feature that’s accessible in out-of-browser mode only is the Notification or ‘toast’ Window, a small area whose maximum size can’t exceed 400x100 pixels that appears for a predefined period of time in screen’s lower right corner and closes automatically when interval expires.
To display a notification window, create a UserControl that will represents notification content, and associate it to NotificationWindow’s Content property as shown in following sample:
NotificationWindow notifyWin = null;
private void OnToastWindowClick(object sender, RoutedEventArgs e)
{
if (App.Current.IsRunningOutOfBrowser)
{
int interval = 3500;
//ToastWindow is a userControl representing notification content
ToastWindow content = new ToastWindow();
if (notifyWin == null)
notifyWin=new NotificationWindow()
{
Content=content,
Width=content.Width,
Height=content.Height
};
else
{
if(notifyWin.Visible) notifyWin.Close();
}
notifyWin.Show(interval);
}
}
trying to show a notification that’s already visible results in an exception, that’s why the sample checks notification’s Visible property and closes current window before invoking Show method again.
Summary
Out-of-browser is one of the most appealing features available in Silverlight since it quickly allows transformation of a web application in a desktop ‘like’ one; Silverlight 4.0 improves it with elevated trust mode that opens the doors to a new generation of RIA applications that can easily run on both Windows and Mac operative systems.