This article is also available in print (Word, PDF) and e-reader formats (MOBI, EPUB).
Guess how many people use Live Services? 500 million plus! And when our applications connect to the same services, they start feeling right at home with what our users are already used to. Microsoft recently opened the
Live Connect APIs, along with some SDKs for 3rd party applications on desktop/web/mobile platforms to leverage the cloud offerings from Live Services.
In this short article, we take a look at how we could get started using the Live Connect API from our Windows Phone applications and interact with SkyDrive storage through the new Live SDK.
[Please note that unlike other Demo solutions, you simply cannot hit F5 to run it. For obvious reasons, I have had to strip out references to my personal SkyDrive; so you would have to get yourself a client ID & make a one-word change before running the solution. Steps explained below.]
Introduction
The new Live APIs expose information around the following .. more information @ Live Connect Developer Guide:
- SkyDrive -- This is for working with files & media; we can read/write against the user's SkyDrive files/folders/albums. SkyDrive offers the perfect cloud storage for Windows Phone applications to push persistence to the user's SkyDrive or to act as back-up storage.
- Hotmail -- These sets of APIs expose the users contacts & calendars for read/writes.
- Messenger -- These APIs are for use in instant messaging. We can read user's current status & communicate with user's buddies.
- Windows Live ID -- This acts as a sort of Access Control Service that we could use to authenticate users & access their Live profile information.
The Live Connect APIs use standard protocols such as HTTP, OAuth 2.0, JSON and XMPP to make it easy working with them across multiple platforms. To leverage the APIs, we primarily use Representational State Transfer (REST) requests which return information in JSON.
While any of the above classes of APIs may be useful from a Windows Phone application, in this article we shall focus on the SkyDrive APIs, which arguably would be the most commonly used. No longer would developers need to spin up storage in cloud to augment their solutions; we can simply leverage the user's SkyDrive which has the additional benefit of user's accessibility & familiarity.
Getting Started
So, let's start integrating the Live Connect SDK into our Windows Phone application:
- While the standard technologies of HTTP/REST/JSon make it easy to work with in any platform, there is some additional help in the form of wrapper SDKs for Windows Phone & Windows 8. Let's grab the Windows Phone Live SDK first.
- Next, to have Live Connect recognize requests from our Windows Phone App, we need an unique identifier, called the Client ID. So, let's head over to the Live Connect App Management site & create a new application to interact with Live Services.
- The new application will be registered with a Client ID, along with a Client Secret & Redirect Domain (that you type in). The two latter ones are not strictly needed for Windows Phone integration. The end result should be something like this:
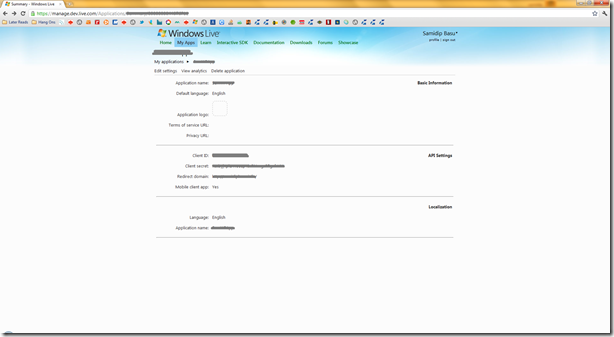
- Live Connect is a combination of several services allowing access to different categories of information. These are organized into what's called Scopes, and explicit declaration & user permission is required for our App to access the categorized information; as a good practice, we should never ask for more than what our App actually needs. For more information, please see Scopes & Permissions.
- Users need to signed in with their Live Credentials & provide consent to utilize their SkyDrive, before we can leverage Live Connect APIs to manipulate files/folders in SkyDrive. While there are several ways to achieve this, in the XAML/C# world of Windows Phone, the easiest is to utilize the built-in Sign-in control in the SDK. Before you use it, make sure to Add Reference to these two libraries:
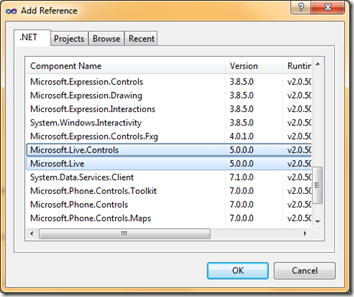
- Next,, if you're in the XAML Designer view & you don't see the Sign-in control in your Toolbox, here's how to add it. The obvious benefit of using the Sign-In control is that we do not need to write the low-level HTTP RESTful GET calls to authenticate & authorize our user; the log-on UI comes up automatically & the client classes hang on to the access tokens passed back from the sign-in control for utilization in future requests.
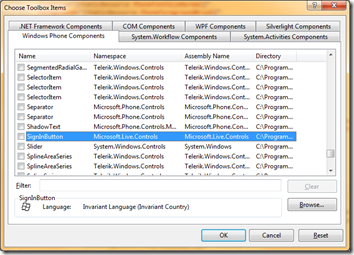
The Demo App
So, let's write a simple Windows Phone application that allows the user to sign in & see/insert files in one's SkyDrive storage. Here's some XAML on our MainPage.xaml to show the sign-in control. Watch the use of scopes to declare what we want access to:
1: <Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
2: <StackPanel Orientation="Vertical" >
3: <TextBlock Text="Please sign-in and allow access to your SkyDrive!" Margin="20" TextWrapping="Wrap" Width="450" FontSize="30"/>
4: <live:SignInButton Name="btnSignin" ClientId="Your_Client_ID" Scopes="wl.signin wl.basic wl.skydrive wl.skydrive_update"
5: RedirectUri="Your_Redirect_URI" Branding="Windows" TextType="SignIn" SessionChanged="btnSignin_SessionChanged"
6: HorizontalAlignment="Center" VerticalAlignment="Top" />
7: <TextBlock x:Name="txtLoginResult" HorizontalAlignment="Center" Margin="0,20,0,0" FontSize="30" Style="{StaticResource PhoneTextAccentStyle}"/>
8: <TextBlock x:Name="txtWelcome" HorizontalAlignment="Center" Margin="0,20,0,0" FontSize="30" Style="{StaticResource PhoneTextAccentStyle}"/>
9: <Button x:Name="btnShowContent" Content="Show File Content" Width="300" Margin="30" Visibility="Collapsed"
10: Click="btnShowContent_Click"/>
11: <Button x:Name="btnAddFile" Content="Add File" Width="300" Margin="30, -20,30,30" Visibility="Collapsed"
12: Click="btnAddFile_Click"/>
13: </StackPanel>
14: </Grid>
The resultant UI before the user signs in as as follows. Also, on first usage of the sign-in button, the user will be prompted to sign-on to Windows Live and provide one-time OAuth access to our application, as demonstrated below:
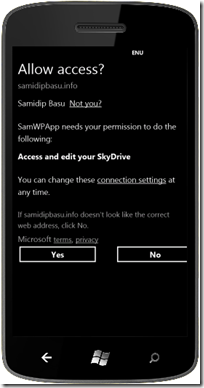
And here's some C# code to handle sign-on attempts. We use the wl.signin to log the user in & wl.basic to greet the user after reading some basic identifying information:
1: private void btnSignin_SessionChanged(object sender, LiveConnectSessionChangedEventArgs e)
2: {
3: if (e.Status == LiveConnectSessionStatus.Connected)
4: {
5: client = new LiveConnectClient(e.Session);
6: App.Current.LiveSession = e.Session;
7: this.txtLoginResult.Text = "Signed in.";
8: this.txtWelcome.Visibility = System.Windows.Visibility.Visible;
9: this.btnShowContent.Visibility = System.Windows.Visibility.Visible;
10: this.btnAddFile.Visibility = System.Windows.Visibility.Visible;
11:
12: client.GetCompleted += new EventHandler<LiveOperationCompletedEventArgs>(OnGetCompleted);
13: client.GetAsync("me", null);
14: }
15: else
16: {
17: this.txtLoginResult.Text = "Not signed in.";
18: this.txtWelcome.Visibility = System.Windows.Visibility.Collapsed;
19: client = null;
20: }
21: }
22:
23: void OnGetCompleted(object sender, LiveOperationCompletedEventArgs e)
24: {
25: if (e.Error == null)
26: {
27: if (e.Result.ContainsKey("first_name") &&
28: e.Result.ContainsKey("last_name"))
29: {
30: if (e.Result["first_name"] != null &&
31: e.Result["last_name"] != null)
32: {
33: this.txtWelcome.Text =
34: "Hello, " +
35: e.Result["first_name"].ToString() + " " +
36: e.Result["last_name"].ToString() + "!";
37: }
38: }
39: else
40: {
41: txtWelcome.Text = "Hello, signed-in user!";
42: }
43: }
44: else
45: {
46: txtWelcome.Text = "Error calling API: " +
47: e.Error.ToString();
48: }
49: }
Did you notice a couple of fun things? Once connected, we store the instance of the LiveConnectClient as a property on our App object; this allows for reuse throughout the application. Also, the RESTful Live APIs support several shortcuts that include “me” to indicate the current user. And here's the UI we are shooting for, after sign-on:
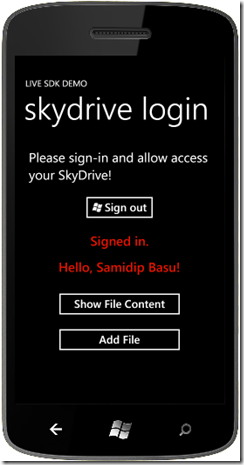
SkyDrive Files
Now that we have permission to user’s Live Services, let’s dig into SkyDrive. Most of us are used to storing documents & media on SkyDrive, and have the content show up in our Windows Phone, or folders kept in sync between multiple PCs/Macs through Live Mesh. With programmatic access to SkyDrive, we have access to a lot of operations, such as:
- Create/Read/Update/Delete Folders
- Do the same with Albums, a special folder to handle media efficiently
- Traversing Directories
- Upload/Download Files
- Read/Update file/folder properties
- Move/copy files/folders etc.
For much more information & details, please see SkyDrive Developer Center. For our Demo application, let us do the simple operations of reading files/folders from user’s SkyDrive root & adding files to it. Here’s how we fetch & list all content from the root directory on user’s SkyDrive:
1: public class SkyDriveContent
2: {
3: public string Name { get; set; }
4: public string Description { get; set; }
5: }
6:
7: // Code in Phone Page.
8: List<SkyDriveContent> ContentList = new List<SkyDriveContent>();
9:
10: private void PhoneApplicationPage_Loaded(object sender, RoutedEventArgs e)
11: {
12: LiveConnectClient client = new LiveConnectClient(App.Current.LiveSession);
13: client.GetCompleted += new EventHandler<LiveOperationCompletedEventArgs>(clientDataFetch_GetCompleted);
14: client.GetAsync("/me/skydrive/files");
15: }
16:
17: void clientDataFetch_GetCompleted(object sender, LiveOperationCompletedEventArgs e)
18: {
19: if (e.Error == null)
20: {
21: List<object> data = (List<object>)e.Result["data"];
22: foreach (IDictionary<string, object> content in data)
23: {
24: SkyDriveContent skyContent = new SkyDriveContent();
25: skyContent.Name = (string)content["name"];
26: ContentList.Add(skyContent);
27: }
28:
29: this.contentList.ItemsSource = ContentList;
30: }
31: }
And, here’s the resulting UI that we bind the results to, simply showing the content names from SkyDrive root:
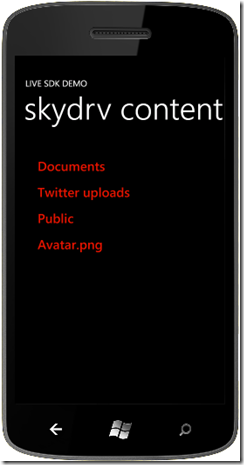
Did you notice how we used “me/skydrive/files” to fetch all content from user’s SkyDrive root? Every folder/album in SkyDrive has a unique ID, visible when we inspect the folder properties. From this point on, we can easily dive into any folder & traverse the user’s SkyDrive by making requests with Folder_ID/files or Album_ID/files. Having fun?
Now, how about putting files in user’s SkyDrive? Sure, we can .. here’s some simple UI & code to add a file to the root of the user’s SkyDrive directory:
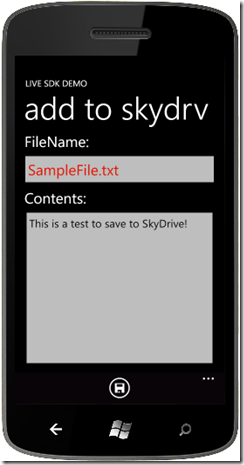
1: private void btnSave_Click(object sender, EventArgs e)
2: {
3: string fileName = this.fileName.Text.Trim();
4: byte[] byteArray = Encoding.Unicode.GetBytes(this.fileContent.Text.Trim());
5: MemoryStream fileStream = new MemoryStream(byteArray);
6:
7: LiveConnectClient uploadClient = new LiveConnectClient(App.Current.LiveSession);
8: uploadClient.UploadCompleted += new EventHandler<LiveOperationCompletedEventArgs>(uploadClient_UploadCompleted);
9: uploadClient.UploadAsync("me/skydrive", fileName, fileStream );
10: }
11:
12: void uploadClient_UploadCompleted(object sender, LiveOperationCompletedEventArgs e)
13: {
14: if (e.Error == null)
15: {
16: Dispatcher.BeginInvoke(() =>
17: {
18: MessageBox.Show("Voila, Saved to the SkyDrive :)", "All Done!", MessageBoxButton.OK);
19: });
20: }
21:
22: this.NavigationService.GoBack();
23: }
So, what did we see? A simple upload location indicator of “me/skydrive” puts the file at the root directory. You can certainly put it in any folder of your choice, as long as you know the ID of the folder. For confirmation, surely authenticate yourself & check if the file addition did work on your SkyDrive. Did for our case
:
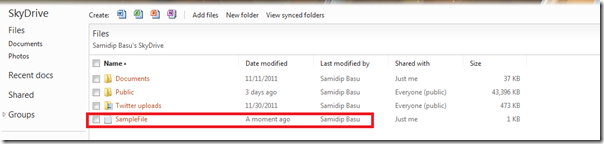
Conclusion & Caveats
- With great power, comes great responsibility! Let us not undermine the end-user’s faith in SkyDrive.
- More specific details about SkyDrive File/Folder APIs can be found HERE.
- Watch the BUILD session recording on Live Connect HERE.
- SkyDrive is a fantastic reliable alternative to augment storage for our Windows Phone applications, and user’s immediately see the file/folders we add in their SkyDrive. More importantly, Office sync on Windows Phone, and the dedicated SkyDrive apps on Windows Phone, iPhone & iPad immediately make the files available to the user. The story gets even better with built-in support in Windows 8, but I digress
- Needless to say, SkyDrive access requires network connectivity. So, this should not possibly be our primary means of storage on Windows Phones.
Summary
In this article, we talked about how to leverage the new Live Connect APIs, in particular, the Live SDK in our Windows Phone applications. We saw the usage of the Sign-in control to ask for user’s authentication & seeking permission to use specific resources through the Live API. SkyDrive access is now handy, with full support to traverse, read & manipulate content in user’s SkyDrive directories. So, what are you waiting for? Look up some documentation & enable SkyDrive access from your Windows Phone application, resulting in happy users & happier you!
I would appreciate any comments or concerns or how things could be done better. Thanks for reading & happy coding.
Cheers SilverlightShow!
About the Author
Samidip Basu (@samidip) is a technologist & gadget-lover working as a Manager & Solutions Lead for Sogeti USA out of the Columbus Unit. With a strong developer background in Microsoft technology stack, he now spends much of his time in spreading the word to discover the full potential of the Windows Phone platform & cloud-backed mobile solutions in general. He passionately runs the Central Ohio Windows Phone User Group (http://cowpug.org), labors in M3 Conf (http://m3conf.com/) organization and can be found with at-least a couple of hobbyist projects at any time. His spare times call for travel and culinary adventures with the wife. Find out more at http://samidipbasu.com.