This article is Part 1 of the series “Producing and Consuming OData in a Silverlight and Windows Phone 7 application.”.
Watch the video tutorial
Download the source code | Download the slides
The Open Data Protocol (OData) is simply an open web protocol for querying and updating data. It allows for the consumer to query the datasource (usually over HTTP) and retrieve the results in Atom, JSON or plain XML format, including pagination, ordering or filtering of the data.
In this series of articles, I am going to show you how to produce an OData Data Source and consume it using Silverlight 4 and Windows Phone 7. Read the complete series of articles to have a deep understanding of OData and how you may use it in your own applications.
Creating our first OData Data Source.
Most OData examples that I’ve seen are using one of the many existing OData Producers. The Netflix catalog is one of the most popular live OData Services that is used in these types of demos. I believe that the best way to teach someone how to use a technology is by building each component starting from File->New Project. I will begin this series by creating an OData producer. In this example, we will build our first OData Data Source by using SQL Server Compact Edition 4.0.
Getting Setup (you will need…)
- Visual Studio 2010 with at least Service Pack 1.
- Microsoft SQL Server Compact 4.0
- Microsoft Visual Studio 2010 SP1 Tools for SQL Server Compact 4.0.
- Microsoft SQL Server Compact 4.0 Tools.
Please note: All of these tools can be acquired using the Web Platform Installer 3.0 available on MSDN.
(screenshot of the Web Platform Installer 3.0 with the SQL components highlighted)
After installing the necessary components, we will begin at File->New Project inside of Visual Studio 2010.
Let’s begin by clicking Web –> ASP.NET Empty Web Application and giving it a Name of “SLShowODataP1”.
Now that our project is created, let’s go ahead and right click on our Solution and add an item.
You will be presented with the “Add New Item” screen. Go ahead and search for the keyword “compact” and Select SQL Server Compact 4.0 Local Database. We will go ahead and give this a name of “Customers.sdf”. Please note: if you do not have the option to select SQL Server Compact 4.0 then you will need to re-read the section titled, “Getting started” above.
After you click on “Add”, you will be presented with the message located below. Go ahead and click “Yes”. This will simply add an “App_Data” folder to our ASP.NET Application.
Now that the local database is created. We will need to right click on it and select Open.
You can now look inside of “Server Explorer” and see your “Customers.sdf” file. Go ahead and right click on Tables and select “Create Table.”
Name the table, “CustomerInfo” and setup the following Columns. (Note: You can look at the image below or follow the column guide below)
- ID – Data Type as int – Primary Key – YES (Make sure to set Identy to True)
- FirstName – Data Type as nvarchar and everything else should be default.
- LastName – Data Type as nvarchar and everything else should be default.
- Address – Data Type as nvarchar and everything else should be default.
- City – Data Type as nvarchar and everything else should be default.
- State – Data Type as nvarchar and everything else should be default.
- Zip – Data Type as nvarchar and everything else should be default.
Now that we have our database setup, lets go ahead and generate some sample data. Right click on CustomerInfo and select “Show Table Data”.
Let’s go ahead and generate some “Fake Data”. I like to use “Fake Name Generator” to generate good sample data. You can use whatever you like. Below is a screenshot of what I used.
Let’s go ahead and create the Service Now. Go back to your project and right click your project and select “Add” –> “New Item”.
Do a search for “entity data model” and select ADO.NET Entity Data Model. Give it a name of “CustomersModel.edmx”.
The following Wizard will be shown on your screen. We are going to take the default option “Generate the model from an existing database” and select “Next”.
The Customers.sdf should be the default data connection on the second screen. If its not select it and leave everything else alone. Go ahead and click “Next”.
We are going to put a check in Tables to select all of our tables (which should only be one). You can leave the other options at their default selection. After you are finished with that click “Finish”.
At this point, our Model has been created by Visual Studio and we are ready to create the oData Service.
Let’s go ahead and create the Service Now. Go back to your project and right click your project and select “Add” –> “New Item”.
Do a search for “data service” and select WCF Data Service. Give it a name of “CustomerService.svc”
After the service is created, we will want to make a few minor tweaks to the supplied code. If you followed my tutorial then you should be able to copy/paste the following code snippet into your CustomerService.svc.cs file. If this doesn’t work then don’t worry you can always scroll to the top of this article and download the complete source code.
1: public class CustomerService : DataService<CustomersEntities>
2: {
3: // This method is called only once to initialize service-wide policies.
4: public static void InitializeService(DataServiceConfiguration config)
5: {
6: config.SetEntitySetAccessRule("CustomerInfoes", EntitySetRights.AllRead);
7: config.DataServiceBehavior.MaxProtocolVersion = DataServiceProtocolVersion.V2;
8: }
9: }
On line 1, you will see that the DataService is the main entry point for an ASP.NET ADO Service. It is expecting a generic that was actually created by Entity Framework called CustomersEntities. This code was generated by Visual Studio and all we have to do is plug it in.
We did not make any modifications to line 4, but on line 6, we added “CustomerInfoes” which was also created by Entity Framework. If you remember from an earlier step, we selected the option to “pluralize” generated object names.This is the reason this field is named “CustomerInfoes”. We also set the Entity rights to full read access. The protocol version on line 7 was set to V2 as this relates to the AtomPub protocol.
Go ahead and right-click the service named, “CustomerService.svc” and select “View in Browser”
Depending on what browser you are using, you should see the following:
(in my case I am using Internet Explorer 8)
URL – “http://localhost:6844/CustomerService.svc/” – Note the localhost:6844 will be different depending on the port number Visual Studio generated for your project.
At this point you have “produced” an OData Data Service. Let’s examine the URI:
This is called a Service Root URI – it simply identifies the root of the oData Service.
We can now navigate one Level up and start to view our “CustomerInfoes” collection. Note: You may have to “View Source” to view the raw xml.
As you can see from the image below, we are seeing our first entry in our collection which is “Michael Crump”
URL - http://localhost:6844/CustomerService.svc/CustomerInfoes
We can now take this same collection and navigate to an individual entry by doing the following:
URL - http://localhost:6844/CustomerService.svc/CustomerInfoes(2)/
As you can see we just selected our second item from the Collection.
Let’s go ahead and create a filter. We only want to show items that the state equal “AL”.
URL - http://localhost:6844/CustomerService.svc/CustomerInfoes?$filter=State eq 'AL'
Let’s take this another step forward and add multiple parameters. Let’s filter by the ID greater than 3 and order the data by FirstName.
URL - http://localhost:6844/CustomerService.svc/CustomerInfoes?$filter=ID gt 3&$orderby=FirstName
Let’s examine the URI now:
To recap:
- The service root URI identifies the root of an OData service.
- The resource path section of a URI identifies the resource to be interacted with (such as Customers, a single Customer, Orders related to Customers in London, and so forth).
- System Query Options are query string parameters a client may specify to control the amount and order of the data that an OData service returns for the resource identified by the URI.
Definitions provided by the official OData URI Conventions documentation.
As you can see there is a lot of possibilities of querying the data within the Web Browser. If you want to look at a complete list then visit the OData site to learn more.
Let’s go ahead and take a look at querying the data using a free tool called “LinqPad”.To get started go ahead and download linqpad at http://linqpad.net/. Once you have it installed, we will need to keep our Visual Studio project running and Add an connection to the OData Service.
You can do this by clicking “Add Connection” –> WCF Data Services (OData) –> Next.
You are going to want to add in your URL to your service on the screen below.
You can leave the username/password fields blank. Now hit OK to continue.
At this point, we are going to run a simple query to our OData Service. So make sure under databases your OData Service is selected. Now type:
from g in CustomerInfoes
select g
You should see the results listed below:
Let’s try one more in order to demonstrate a where and orderby clause.
Now type:
(from g in CustomerInfoes
where g.ID > 2
orderby g.FirstName
select g)
Conclusion
At this point, we have seen how you would produce an OData Data Source and learn some basic sorting and filtering using the web browser and LinqPad. In the next part of the series, I am going to show you how to consume this data in a Silverlight Application and in Part 3 a Windows Phone 7 Application. Again, thanks for reading and please come back for the final two parts.
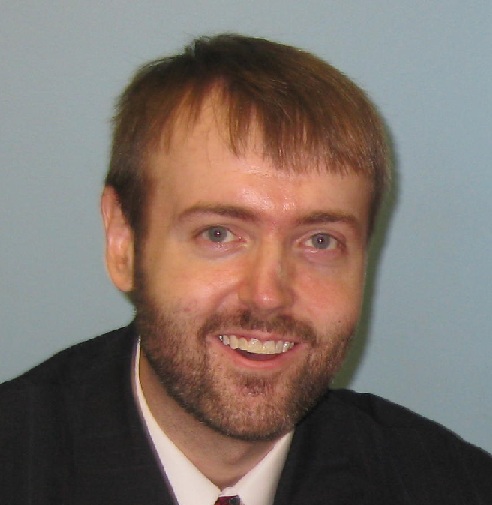
Michael Crump is an MCPD that has been involved with computers in one way or another for as long as he can remember, but started professionally in 2002. After spending years working as a systems administrator/tech support analyst, Michael branched out and started developing internal utilities that automated repetitive tasks and freed up full-time employees. From there, he was offered a job working at McKesson corporation and has been working with some form of .NET and VB/C# since 2003.
He shares his findings in his personal blog: http://michaelcrump.net and he also tweets at:@mbcrump