This article is compatible with the latest version of Silverlight for Windows Phone 7.
At the end of the previous part of this series we created a very simple application, starting from the Visual Studio template, at the sole scope of showing how simple is to create a Silverlight project and having it deployed to the phone for development purposes. We easily ran the Hallo Windows Phone application directly from the IDE and debugged it as we are habit with all the other common project types.
If we go deep inside the project structure we encounter some little differences from a normal Silverlight project made for the desktop. At the first sight it can seem almost the same, but there are some phone-specific details we need to know. So let me explore them briefly just before speaking on the structure of the page and of the application itself.
This is the planned Table of Contents for the whole series. If you recommend adding another topic to the TOC - please feel free to comment below the article.
Windows Phone 7 Part #1: Getting Started
Windows Phone 7 Part #2: Your first app
Windows Phone 7 Part #3: Understanding navigation
Windows Phone 7 Part #4: The application lifecycle
Windows Phone 7 Part #5: Panorama and Pivot controls
Windows Phone 7 Part #6: Advanced interaction
Windows Phone 7 Part #7: Understanding Push Notifications
Windows Phone 7 Part #8: Using Sensors
WPAppManifest.xml and App.xaml
Once you analyze the project you have just created, a couple of things become immediately evident. Also if you have a MainPage.xaml, an App.xaml and a Properties folder like a common Silverlight project, there are also a number of image files that may seems strange. But the very big differences are inside the App.xaml.cs file (the codebehind for the application) and in a file called WPAppManifest.xml that is located inside the Properties folder.
The code inside of the codebehid of the application is so far different from a common Silverlight application. The reason of this difference is to be found in the structure of a Windows Phone application. Differently from Silverlight, every application for the phone is a Navigation Application, so, the root visual is automatically set to a PhoneApplicationFrame inside of this file. Even if you are able to manually remove this code you are not allowed to do it, because setting the RootVisual to something different from this control will cause the O.S. watchdogs drops your application because it does not perform any navigation. Additionally if you don't use this control you will not be aware of what the user is doing with the navigation buttons.
The most important file is the WPAppManifest.xml that is the central point where they reside the metadata of the software. Inside this file, that you usually edit using the Properties Window, but sometimes it is required you change by hand, there is some things that really matter. You may find the name of the startup page, usually set to MainPage.xaml, you may find the Title, the author informations and the Product ID for your app. Also there is a section called PrimaryToken that describes the aspect of the Tile when the application is docked to the main phone menu. Many of the properties refer to the images you may find inside the root of the project. Here is the meaning of any image:
ApplicationIcon.png |
The icon displayed by the phone in the start menu |
Background.png
|
The background of the tile showed when the application is docked to the main screen. This image can be changed only when a push notification is issued |
SplashScreenImage.jpg
|
The image showed during the first phase of the lifecycle of the application while the runtime is loading it. |
One important note about a section of this file is related to the phone "capabilities". This section contains a series of tags that refers to what the application requires to run. The capabilities are a model that enables certain features of the phone only when you are aware you need them. It is important to know this section, because during the submission process of the XAP, a MSIL analisys tool is used to detect the capabilities your application needs to run, and the content of the file is changed according to the result of the test. So the best is that you manually try to set this section, to the capabilities you know you are using, to test if they suffice to run without exceptions. Here is an explanation of the capabilities taken directly from MSDN:
Capability ID/Name
|
Description
|
ID_CAP_NETWORKING
|
Applications with access to network services. This must be disclosed because services can incur cost when a phone is roaming.
|
ID_CAP_IDENTITY_DEVICE
|
Applications that use device-specific information such as a unique device ID, manufacturer name, or model name.
|
ID_CAP_IDENTITY_USER
|
Applications using the anonymous LiveID to uniquely identify the user in an anonymous fashion.
|
ID_CAP_LOCATION
|
Applications with access to location services.
|
ID_CAP_SENSORS
|
Applications using the Windows Phone sensors.
|
ID_CAP_MICROPHONE
|
Applications that use the microphone. The application can record without visual indication that recording is taking place.
|
ID_CAP_MEDIALIB
|
Applications that can access the media library.
|
ID_CAP_GAMERSERVICES
|
Applications that can interact with Xbox LIVE APIs. This must be disclosed due to privacy issues since data is shared with Xbox.
|
ID_CAP_PHONEDIALER
|
Applications that can place phone calls. This may happen without visual indication for the end user.
|
ID_CAP_PUSH_NOTIFICATION
|
Applications that can receive push notifications from an Internet service. This must be disclosed as usage could incur roaming charges.
|
ID_CAP_WEBBROWSERCOMPONENT
|
Applications that use the web browser component. There are security risks with scripting.
|
Just remember that, if a capability is switched off removing the corresponding tag from the section, the runtime will raise and exception if you try to use the feature.
The first contact with the UI
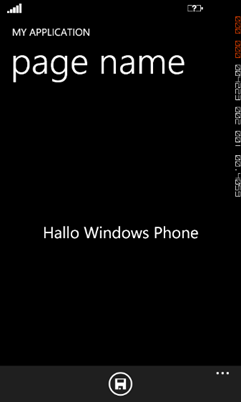
Once you start building your applicaton the very first thing you have to do is configuring the page to meet your requirements. As you can see on the right side, the page have some different parts you need to know. Every part has a specific function you can decide to switch it on or off to follow your will. At the very top of the page there is the System Tray where you see the battery meter and the signal strenght but it can contain a lot of other infos like the wi-fi status and so on. This part may be hidden if your application need to use this space for its purposes. You have simply to set a property on the PhoneApplicationPage control:
<phone:PhoneApplicationPage
x:Class="WindowsPhoneApplication1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
shell:SystemTray.IsVisible="False">
</phone:PhoneApplicationPage>
On the bottom side of the page there is the ApplicationBar. This component can display a number of circular buttons with a small icon and if you use Blend you will find an useful collection of predefined graphics to use in your applications. But the ApplicationBar is also host for a number of menu items that are displayed once you click on the three dots on the right.
If you need this space you can simply avoid to insert a section in the XAML but I think it is so useful and well known by phone's users that the most of the times you will leave it available and with a bunch of commands inside. In the following box you may see an example of ApplicationBar with a button (Save) and two MenuItems (Settings, About).
<phone:PhoneApplicationPage.ApplicationBar>
<shell:ApplicationBar IsVisible="True" IsMenuEnabled="True">
<shell:ApplicationBarIconButton IconUri="/icons/appbar.save.rest.png" Text="Save"/>
<shell:ApplicationBar.MenuItems>
<shell:ApplicationBarMenuItem Text="Settings..."/>
<shell:ApplicationBarMenuItem Text="About..."/>
</shell:ApplicationBar.MenuItems>
</shell:ApplicationBar>
</phone:PhoneApplicationPage.ApplicationBar>
It is important to be aware that the ApplicationBar is not a Control. This leads to a couple of common errors when you start the first time. First of all being not a Control means that the FindName method is not able to find it inside the VisualTree so, also if you give it an "x:Name" the reference in the codebehind is always null. Additionally the properties of the ApplicationBar are not DependencyProperties so for example the Binding markup extension will not work.
Finally, as you can see in the picture, on the right side of the screen there is a series of numbers. These are counters related to the performances of the UI and are useful when you are debugging animation-intensive UI where being aware of the frame rate is something important. Usually these counters are enabled only when you compile in debug but if you do not want them you can set to False this property in the App.xaml.cs:
Application.Current.Host.Settings.EnableFrameRateCounter = false;
Dealing with orientation
Depending on how your application works and what you are presenting to the user, you can choose to present your UI in vertical (Portrait) or in horizontal (Lanscape). You have to consider that for the most of the time the user will manipulate his phone in Portrait mode. This is the most obvious way to use the phone because all the buttons and the shape of the device are designed with this in mind. But for sure there are many cases when, the presented UI, gets a big advantage from the Landscape mode, for example when you are projecting videos or images in full screen. When you are planning the content of a page you can choose between three supported orientations; You can fix the page to Portrait or Landscape or you may give the choice to the user setting the SupportedOrientation property to PortraitOrLandscape:
<phone:PhoneApplicationPage
x:Class="WindowsPhoneApplication1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
SupportedOrientations="PortraitOrLandscape" Orientation="Portrait">
</phone:PhoneApplicationPage>
As you might have understand the SupportedOrientation property sets the orientation your application will support and the Orientation property sets the default orientation that the page will have at the startup. As an example if you set both the SupportedOrientation and Orientation to "Landscape" the page will use this orientation and does not give the user the ability to change it.
If you support both the orientation you will be notified when the user change it from portrait to lanscape of from landscape to portrait. To be notified about this matter you can subscribe the event OrientationChanged in the PhoneApplicationPage control and you will get an event raised every time the switch occur. There are many times when you do not need to handle this event. Setting up the UI with this in mind can take advantage of the automatic handling of the orientation that most of the controls implement.
The trick is that when the orientation changes, the layout and all the content will automatically switch the vertical dimension with the horizontal so the layout is automatically updated to reflect this change. For your code it will be the same that the user has suddenly resized the page from 480x800 to 800x480. So you really need to be aware of the new orientation only if you decide of completely change the layout to better use the space of the page.In other cases this event may be not useful.
The Metro Theme
When you meet your phone for the first time, the first thing it becomes evident is the user interface theme that is the distinctive element from your Windows Phone and other O.S. brands. This theme has been well designed to make your device usable and friendly. Starting from Tiles, to every graphical element it permeate all the device interface. Also, when you explore the settings menu you meet the theme configuration that lets you switch between the default dark theme and the light theme that is more or less the inverse of the other, where the background appears light and the text become dark.
These themes is reflected in any application you will write for the Windows Phone 7, and you have to pay lot of attention to them, because a part of the certification process directly involve the support to both the phone themes. If you does not test your application with both the themes, it's easy your submission will be rejected because of parts of the UI that are non visible with a theme or the other.
To help you with this work, the runtime will initialize a set of theme resources you can find detailed here, that simplify the process of apply default themes to your application's UI. The metro resources includes lot of colors, brushes, fonts, and all the default templates of Windows Phone controls rely on them to depict their aspect. The resources are automatically added to the Application Resources collection and become accessible both from the XAML or the codebehind. The wizard itself will automatically add some references to them for the Page fonts and Blend will give you the full set of resources to apply to the various elements.
In the case the theme resources does not help you in the process remember that there is 4 resources named PhoneDarkThemeVisibility, PhoneLightThemeVisibility, PhoneDarkThemeOpacity and PhoneLightThemeOpacity that help you hide or show different partes of the interface when the themes are applied.
Finally, not always the default theme resources have the colors, the size and the fonts you want for your interface. In these cases the best is to redefine the theme resources with your own characteristics. It may be achieved adding the new definition to the App.xaml file with the same name and all the elements will reflect this change. Remember that if you need to change the colors you have to redefine the brush resources because color resources are not overridable.
An example to better understand
Since we have talked about many details, I've prepared a simple project that uses some of the concepts I've explained.
Download Code
Once you download and run, it shows a sort of gameboard with an ApplicationBar with buttons and menu items. It handles the orientation changes and use the theme resources to make the UI adaptive to the theme. In the App.xaml you may find a chunk of markup where if you remove the comment redefine some resources.
From the basics we have put in place in this article, in the next appointment we will start to build something more complicated, with more than a single page.
About the Author
Andrea Boschin is 41 years old from Italy and currently lives and works in Treviso, a beautiful town near Venice. He started to work in the IT relatively late after doing some various jobs like graphic designer and school teacher. Finally he started to work into the web and learned by himself to program in VB and ASP and later in C# and ASP.NET. Since the start of his work, Andrea found he likes to learn new technologies and take them into the real world. This happened with ASP.NET, the source of his first two MVP awards, and recently with Silverlight, that he started to use from the v1.0 in some real projects.