This article is sponsored by
Telerik RadControls for Silverlight. For similarly awesome content check out
Telerik XAMLflix, your step-by-step guide to Telerik Silverlight and WPF controls. Get access to video tutorials, written tutorials, and tons of code!
To contact me directly please visit my blog at http://michaelcrump.net/ or through twitter at http://twitter.com/mbcrump.
This article is Part 8 of the series “10 Laps around Silverlight 5.” If you have missed any other section then please see the Roadmap below.
To refresh your memory on what Silverlight is:
Microsoft Silverlight is an application framework for writing Rich Internet Applications.
The run-time environment is available as a plug-in for most web browsers and works on a variety of operating systems including Windows, Mac and Linux.
To recap what we learned in the previous section:
- We learned how you could specify a default filename when using the SaveFileDialog prompt. We also discussed how you could run the application in elevated trust to prevent the security warning from being displayed.
- We then took a look at 64-bit browser support that is included with Silverlight 5 and learned that if your user is using a 64-bit browser then they will be redirected to a location to download the Silverlight 5 x64 runtime.
- We wrapped up a short section on new and improved power awareness for media applications.
In this article, I am going to discuss productivity and performance enhancements in Silverlight 5 including: XAML Binding Debugging, Parser Performance Improvements and Multi-core JIT for improved start-up time. Please review the Roadmap for the series before going any further.
The Roadmap for this Series
I’ve included the Roadmap for the series below as you may want to visit other sections as you learn Silverlight 5. I picked the following features as I thought that you may find them useful in your day-to-day work. If you want a specific topic covered then please leave it in the comments below.
1) Introduction to SL5 – This post which provides a brief history of Silverlight and relevant links.
2) Binding- Ancestor Relative Source Binding and Implicit Data Templates.
3) Graphics –XNA 3D API and Improved Graphics Stack.
4) Media - Low-Latency Sound using XNA and Remote Control and Media Command (Keys) Support.
5) Text - Text Tracking and Leading, Linked and Multi-column Text, OpenType Support, Pixel Snapped Text and TextOptions.
6) Operating System Integration - Part 1 - P/Invoke, Multiple Windows and Unrestricted File System Access in Full Trust.
7) Operating System Integration - Part 2 - Default Filename for SaveFileDialog, 64-bit browser support and Power Awareness.
8) Productivity and Performance [This post] - XAML Binding Debugging, Parser Performance Improvements and Multi-core JIT for improved start-up time.
9) Controls - Double and Triple click support, PivotViewer and ComboBox Type-Ahead.
10) Other items - In-Browser HTML, PostScript and Tasks for TPL.
XAML Binding Debugging
XAML Binding Debugging, is one of the most important features in the Silverlight 5. We have all been stuck with Binding expressions at one point or another and wanted an easier way to debug them. Now anywhere that you see a {Binding} expression you can put a break point on it just like your typical C# code. Let’s take a look at a sample:
Fire up a new Silverlight 5 project and give it any name that you want.
Go ahead and add a new class to the project named Podcast.cs and add the following code.
1: public class Podcast
2: {
3: public string Description { get; set; }
4: public DateTime ReleaseDate { get; set; }
5: public Uri Link { get; set; }
6:
7: public Podcast(string description, DateTime releasedate, Uri link)
8: {
9: Description = description;
10: ReleaseDate = releasedate;
11: Link = link;
12: }
13: }
Let’s switch back over to the MainPage.xaml.cs and add the following code:
1: public MainPage()
2: {
3: InitializeComponent();
4: Loaded += new RoutedEventHandler(MainPage_Loaded);
5: }
6:
7: void MainPage_Loaded(object sender, RoutedEventArgs e)
8: {
9: this.DataContext =
10: new Podcast("This Developer's Life - Criticism",
11: new DateTime(2011, 4, 21),
12: new Uri("http://thisdeveloperslife.com/post/2-0-1-criticism", UriKind.Absolute)
13: );
14:
15: }
Switch back over to the MainPage.xaml and add in the following code replacing the current Grid:
1: <Grid x:Name="LayoutRoot" Background="White">
2: <StackPanel Orientation="Vertical">
3: <TextBlock Text="{Binding Description}" />
4: <TextBlock Name="txtReleaseDate" Text="{Binding ReleaseDate}" />
5: <HyperlinkButton Content="Listen to this Episode" NavigateUri="{Binding Lik}" TargetName="_blank" />
6: </StackPanel>
7: </Grid>
While here, go ahead and put a break point on the “Hyperlink" button line, which you can do by clicking outside its margin as shown below:
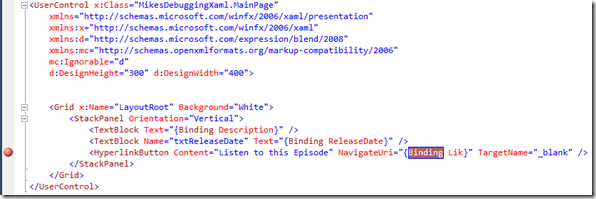
Notice the Red Circle and the highlighted “Binding” word? The Visual Studio 2010 debugger will stop once the XAML parser hits that line.
Please note that if you try to put a breakpoint on any line outside of the “Binding” keyword, then you will get a message saying, “This is not a valid location for a breakpoint” at the bottom of your screen:
We are now ready to begin debugging, so, press “F5” on your keyboard to begin. You will notice that the Binding breakpoint was hit. If you examine your Locals window then you will see the following:
It looks like we have an error in our Binding statement that is telling us the property ‘Lik’ does not exist on the class called Podcast. If you continue running the application then you will quickly notice that the webpage launches but the “Listen to this Episode” hyperlink does not work.
If we take a quick look at our Podcast class then we will notice that the property is actually called Link. If we go back to our MainPage.xaml and change
1: <HyperlinkButton Content="Listen to this Episode" NavigateUri="{Binding Lik}" TargetName="_blank" />
to
1: <HyperlinkButton Content="Listen to this Episode" NavigateUri="{Binding Link}" TargetName="_blank" />
and run our application again it will work properly.
It is very easy to debug Binding expressions now with that feature. Another undocumented feature is that if you have Silverlight 5 installed then you can also debug your Silverlight 4 binding statements! Sweet!
Productivity and Performance Improvements
Silverlight 5 contains many additional performance improvements that you may not be aware of. I have decided to create a short list here:
- Reduced network latency by using a background thread for networking.
- Hardware acceleration is enabled in windowless mode with Internet Explorer 9.
- XAML parser improvements that speed up startup and runtime performance.
- Support for 64-bit operating systems as discussed in part 7 of this series.
- Multi-core JIT for improved startup time.
- Increased performance of hardware decoding and presentation of H.264 media for lower-power devices.
- Text layout performance improvements
As you can see the Silverlight Team has been hard at work not only adding additional features to Silverlight 5 but improving performance all around.
Conclusion
At this point, we have seen how you would debug binding statements using Silverlight 5’s built-in XAML debugger. We also looked at several productivity and performance improvements in Silverlight 5. In the next part of the series, I am going to take a look at several new controls/features shipping with Silverlight 5 including : Double and Triple click support, PivotViewer and ComboBox Type-Ahead. Again, thanks for reading and please come back for the next part.
To contact me directly please visit my blog at http://michaelcrump.net/ or through twitter at http://twitter.com/mbcrump.