This article is sponsored by
Telerik RadControls for Silverlight. For similarly awesome content check out
Telerik XAMLflix, your step-by-step guide to Telerik Silverlight and WPF controls. Get access to video tutorials, written tutorials, and tons of code!
To contact me directly please visit my blog at http://michaelcrump.net/ or through twitter at http://twitter.com/mbcrump.
This article is Part 9 of the series “10 Laps around Silverlight 5.” If you have missed any other section then please see the Roadmap below.
To refresh your memory on what Silverlight is:
Microsoft Silverlight is an application framework for writing Rich Internet Applications.
The run-time environment is available as a plug-in for most web browsers and works on a variety of operating systems including Windows, Mac and Linux.
To recap what we learned in the previous section:
- We learned how you could specify a break point inside of a Binding Expressions in Silverlight 5. We also learned how you could use this same functionality inside of Silverlight 4 with the Silverlight 5 SDK installed.
- We then created a sample application that contained a binding error and took a look at the output window to investigate it further. We were then able to determine the source of our error and correct it quickly.
- We finished up with listing several productivity and performance improvements in Silverlight 5 that you may not be aware of.
In this article, I am going to discuss several new features/controls such as Double and Triple click support, PivotViewer and ComboBox Type-Ahead. Please review the Roadmap for the series before going any further.
The Roadmap for this Series
I’ve included the Roadmap for the series below as you may want to visit other sections as you learn Silverlight 5. I picked the following features as I thought that you may find them useful in your day-to-day work. If you want a specific topic covered then please leave it in the comments below.
1) Introduction to SL5 – This post which provides a brief history of Silverlight and relevant links.
2) Binding- Ancestor Relative Source Binding and Implicit Data Templates.
3) Graphics –XNA 3D API and Improved Graphics Stack.
4) Media - Low-Latency Sound using XNA and Remote Control and Media Command (Keys) Support.
5) Text - Text Tracking and Leading, Linked and Multi-column Text, OpenType Support, Pixel Snapped Text and TextOptions.
6) Operating System Integration - Part 1 - P/Invoke, Multiple Windows and Unrestricted File System Access in Full Trust.
7) Operating System Integration - Part 2 - Default Filename for SaveFileDialog, 64-bit browser support and Power Awareness.
8) Productivity and Performance - XAML Binding Debugging, Parser Performance Improvements and Multi-core JIT for improved start-up time.
9) Controls [This post] - Double and Triple click support, PivotViewer and ComboBox Type-Ahead.
10) Other items - In-Browser HTML, PostScript and Tasks for TPL.
Double and Triple Click Support
One of the new features in Silverlight 5 is the ability to use Double and Triple Click Support. This functionality will tell you how many times the user has clicked the mouse button. The property is called ClickCount and resides in the MouseButtonEventArgs class. Let’s take a look at how to use this new feature.
Fire up a new Silverlight 5 project and give it any name that you want.
Switch over to the MainPage.xaml.cs and add the following code: (Note: You may not need the MainPage() Method section)
1: public MainPage()
2: {
3: InitializeComponent();
4: }
5:
6: private void textBlock1_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
7: {
8: textBlock1.Text = e.ClickCount.ToString();
9: }
Switch back over to the MainPage.xaml and add in the following code replacing the current Grid:
1: <Grid x:Name="LayoutRoot" Background="White">
2: <Border BorderBrush="Black" BorderThickness="1" Margin="52,49,68,74" CornerRadius="10">
3: <TextBlock Height="152" HorizontalAlignment="Center" x:Name="textBlock1" Text="0" VerticalAlignment="Center" Width="244" MouseLeftButtonDown="textBlock1_MouseLeftButtonDown" Foreground="#FFFF2E2E" FontSize="96" TextAlignment="Center" />
4: </Border>
5: </Grid>
If we go ahead and run the application then we will see the following application.
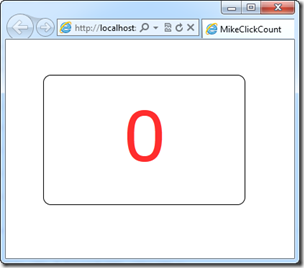
Go ahead and begin clicking inside of the border and you will see the number increase. If you wait a few seconds and click again then you will notice that it reset itself. You could easily add If..Then… statements to determine what click count number they are on. This may be helpful for a 35 click Easter egg. :)
PivotViewer
Microsoft’s Silverlight Team defines it as the following: The Silverlight PivotViewer makes it easier to interact with massive amounts of data on the web in ways that are powerful, informative and valuable. I couldn’t agree more. Let’s begin today by creating a SL5 application that uses the PivotViewer control and displays a collection of movies. (Classic example, eh?)
Please note that this sample barely scratches the surface of what you can do with the PivotViewer. I’ve included an official link by Microsoft at the bottom of this post for your reference.
Fire up a new Silverlight 5 project and give it the name PivotViewer.
Switch over to the MainPage.xaml.cs and add the following code:
1: using System;
2: using System.Linq;
3: using System.Windows;
4: using System.Windows.Controls;
5: using System.Collections.ObjectModel;
6:
7: namespace PivotViewer
8: {
9: public partial class MainPage : UserControl
10: {
11: private ObservableCollection<Movie> Movies;
12:
13: public MainPage()
14: {
15: InitializeComponent();
16: Movies = new ObservableCollection<Movie>();
17: for (Int64 i = 0; i < 500; i++)
18: {
19: Movies.Add(new Movie() { Title = "Ice Age" + i.ToString(), CountryOfOrigin = "USA", Description = "Set during the Ice Age.", Director = "Chris Wedge" + i.ToString(), Duration = 90, ReleaseDate = new DateTime(2002, 09, 03) });
20:
21: }
22: }
23:
24: private void UserControl_Loaded(object sender, RoutedEventArgs e)
25: {
26: MoviePivot.ItemsSource = Movies;
27: }
28: }
29: }
We need to create a new class now named
Movie. Inside of that class should look like the following:
1: using System;
2:
3: namespace PivotViewer
4: {
5: public class Movie
6: {
7: public string Title { get; set; }
8: public int Duration { get; set; }
9: public DateTime ReleaseDate{ get; set; }
10: public string CountryOfOrigin { get; set; }
11: public string Description { get; set; }
12: public string Director { get; set; }
13: }
14: }
Switch back over to the MainPage.xaml and add in the following code replacing everything:
1: <UserControl x:Class="PivotViewer.MainPage"
2: xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
3: xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
4: xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
5: xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
6: xmlns:pivot="clr-namespace:System.Windows.Controls.Pivot;assembly=System.Windows.Controls.Pivot"
7: Loaded="UserControl_Loaded"
8: mc:Ignorable="d"
9: d:DesignHeight="300" d:DesignWidth="400">
10:
11: <Grid x:Name="LayoutRoot" Background="White">
12: <pivot:PivotViewer x:Name="MoviePivot"
13: AccentColor="LightGreen"
14: Background="LightGray"
15: ControlBackground="LightYellow"
16: SecondaryBackground="AntiqueWhite"
17: SecondaryForeground="Bisque"
18: SecondaryItemValueBackgroundColor="Chocolate" >
19:
20: <!--Setting PivotProperties-->
21: <pivot:PivotViewer.PivotProperties>
22: <pivot:PivotViewerStringProperty Id="FTitle" Options="CanFilter" DisplayName="Title" Binding="{Binding Title}" />
23: <pivot:PivotViewerStringProperty Id="FDuration" Options="CanFilter" DisplayName="Duration" Binding="{Binding Duration}" />
24: <pivot:PivotViewerStringProperty Id="FDirector" Options="CanFilter" DisplayName="Director" Binding="{Binding Director}" />
25:
26: </pivot:PivotViewer.PivotProperties>
27:
28: <!--Setting data-->
29: <pivot:PivotViewer.ItemTemplates>
30: <pivot:PivotViewerItemTemplate>
31: <Border Width="200" Height="200" Background="CadetBlue">
32: <StackPanel Orientation="Vertical">
33:
34: <StackPanel Orientation="Horizontal">
35: <TextBlock Text="{Binding Title}" FontSize="16" Foreground="White" />
36: <TextBlock Text="(" FontSize="16" Foreground="White" />
37: <TextBlock Text="{Binding Duration}" FontSize="16" Foreground="White" />
38: <TextBlock Text=")" FontSize="16" Foreground="White" />
39: </StackPanel>
40: <TextBlock Text="{Binding Director}" FontSize="16" Foreground="White" />
41: <TextBlock Text="{Binding CountryOfOrigin}" FontSize="16" Foreground="White" />
42: </StackPanel>
43: </Border>
44: </pivot:PivotViewerItemTemplate>
45: </pivot:PivotViewer.ItemTemplates>
46: </pivot:PivotViewer>
47: </Grid>
48: </UserControl>
If we run our application and select a few items then we will see the following screen. As you can tell when the application loaded that it contained 500 items – Wow!
I’ve barely touched on the amount of things that you can do with PivotViewer. If you want to learn more than please visit the Silverlight site located here.
Combo-Box Type Ahead
Another new feature in Silverlight 5 is Combo-Box Type Ahead. This makes choosing items from a long list very simple. Let’s go ahead and build a sample application.
Fire up a new Silverlight 5 project and give it any name that want.
Switch over to the MainPage.xaml.cs and add the following code:
1: public MainPage()
2: {
3: InitializeComponent();
4: var lstDevelopers = new List<string>
5: { "Michael Crump",
6: "Pete Brown",
7: "Victor G.",
8: "Scott Hanselman",
9: "Jesse Liberty",
10: "Shawn Wildermuth",
11: "Scott Gu",
12: "Joel Cochran",
13: "Kunal Chowdhurry" };
14: cbDevelopers.ItemsSource = lstDevelopers;
15: }
Switch back over to the MainPage.xaml and add in the following code replacing the existing Grid:
1: <Grid x:Name="LayoutRoot" Background="White">
2: <ComboBox x:Name="cbDevelopers" Height="40" Width="150" Margin="20" />
3: </Grid>
If we run our application and begin typing then we will see the ComboBox automatically highlights the row that matches our selection.
Conclusion
At this point, we have seen how you would use Double and Triple click support, PivotViewer and ComboBox Type-Ahead. In the next and final part of the series, I am going to take a look at several new features shipping with Silverlight 5 including : In-Browser HTML, PostScript and Tasks for TPL. Again, thanks for reading and please come back for the next part.
To contact me directly please visit my blog at http://michaelcrump.net/ or through twitter at http://twitter.com/mbcrump.