Hello and welcome to part 3 of our exploration of new developer features in Windows 8.1! In this third part, we will continue looking at some new additions and changes to the WinRT library, the base class library for Windows 8 development. Just to recap, we already covered some XAML stuff and the RenderTargetBitmap class in the previous article. There are some really interesting classes in WinRT that enable new app scenarios and that’s the focus of this article. Among others, we will cover speech synthesis, the new HTTP API and the Contact Manager API.
The new HTTP API
For their data needs, Windows 8 applications are required to work with services. Apart from a SQLite implementation, Windows 8 apps have no local DB access (unless we build one on top of XML files). For up-to-date date however, we have to resort to using services. Windows 8 has quite a few options in this area, including WCF, REST, RSS and much more.
REST (XML or JSON based) services are very popular nowadays. Many web APIs are using it to expose their functionality. In Windows 8, we had the System.Net namespace which contained the HttpClient class (which replaced the WebClient class we had previously in Windows 8). With Windows 8.1, we are getting a new version of the HttpClient, which now lives entirely in WinRT, the Windows.Web.Http.HttpClient (and related classes in Windows.Web.Http). In terms of functionality, the API is more powerful than the one it is replacing; in terms of API, it’s still pretty similar to the old one. Some of the extra functions that are supported in this new version are caching support, access to cookies from the code (wasn’t possible with the old API) and filters.
In the first demo, we’ll use the HttpClient and ask it to load a file from the cache if available. Initially, we’re making the call to the network resource, as shown below.
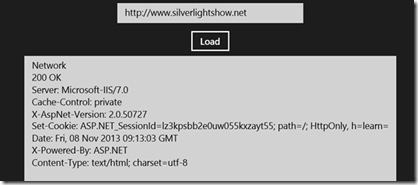
After this call, the page is cached by the system. Subsequent calls can now be served from cache.
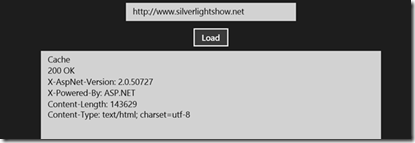
Let’s see how we’ve made these call using code. We’re using an HttpClient and the HttpBaseProtocolFilter. The latter is used to configure how the call is made using the HttpClient instance so it’s this filter that we use to specify we want to use the default cache behaviour. On the new HttpClient, we can use the same methods to get a network resource, in this case, the GetAsyns. After we’ve received the response in the form of an HttpResponseMessage (again the same class as with the “old” HttpClient), we can use the Source property to figure out if we got the cached version of a new instance.
1: private HttpBaseProtocolFilter filter;
2: private HttpClient httpClient;
3:
4: public MainPage()
5: {
6: this.InitializeComponent();
7:
8: filter = new HttpBaseProtocolFilter();
9: httpClient = new HttpClient(filter);
10: //Let's use default cache behavior --> always used cached version
11: filter.CacheControl.ReadBehavior = HttpCacheReadBehavior.Default;
12:
13: }
14: …
15: HttpResponseMessage response = await httpClient.GetAsync(new Uri(AddressTextBox.Text));
16: string result = string.Empty;
17: result = response.Source + Environment.NewLine;
18: result += SerializeHeaders(response);
19:
20: ResultTextBox.Text = result;
If we now want to get access to the cookies that were sent to the device when making the request, we can again use the HttpClient class which now gives access to this collection. In the screenshot below, we can see the cookies being read.
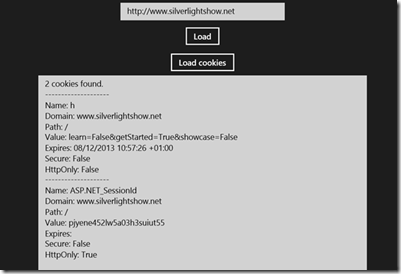
Getting access to the cookies is done using the following lines of code. On the filter, we use the HttpCookieManager, which returns us a collection of cookies associated with the request.
1: HttpBaseProtocolFilter filter = new HttpBaseProtocolFilter();
2: HttpCookieCollection cookieCollection =
3: filter.CookieManager.GetCookies(new Uri(AddressTextBox.Text));
The HttpClient has more options, including for example the RetryFilter. This is handy if the server replies with a 503 including a Retry-After header, which will then indicate to the HttpClient that the request is to be retried after the indicated amount of time. As you can understand, this new API gives us many more options built-in, allowing us to focus on the business-side of things, instead of the technical stuff surrounding the actual creation of the requests.
Speech Synthesis from a WinRT app
Another interesting new feature in Windows 8.1 is speech synthesis. As the name implies, this enables a Windows 8.1 application to read out text to the user. While this may not seem like a big deal, there are a number of interesting scenarios possible with this:
- Turn-by-turn navigation applications can now use the built-in speech synthesis capabilities to read out instructions
- An ebook application can read out the book contents instead of the user having to read the book
- Prompt the user for input
Another interesting aspect is accessibility of your application. If you want to build an app that makes it easy for people with accessibility issues to work with your app and have it read out the names of the input fields for the user. This is certainly an interesting aspect since in some countries, government-owned software and sites need to be conform certain rules regarding accessibility.
The entire concept works based on the classes available in the Windows.Media.SpeechSynthesis namespace. Basically, what happens is that we can build up a stream by reading out text and that stream will then be the source for a MediaElement that will then read out the contents of the stream. Let’s take a look.
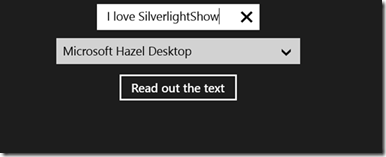
Windows 8 comes with a number of built-in voices, based on the installed languages. On my device, there are 3 voices installed (because of my langauge settings).
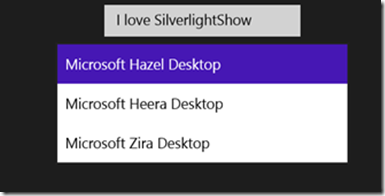
The 2 main classes that enable this are the SpeechSynthesizer and the SpeechSynthesisStream. We have to use the SpeechSynthesizer, assign it a voice to use and pass it the text it needs to read out. That returns a SpeechSynthesisStream which in turn can be set as the source for the MediaElement that needs to read out the contents.
In the code below, we do the first part: we assign the voice to use and also fill the ComboBox with all available voices.
1: speechSynthesizer = new SpeechSynthesizer();
2: this.InitializeComponent();
3:
4: var allVoices = Windows.Media.SpeechSynthesis.SpeechSynthesizer.AllVoices;
5:
6: VoiceInformation currentVoice = this.speechSynthesizer.Voice;
7:
8: foreach (VoiceInformation voice in allVoices)
9: {
10: ComboBoxItem item = new ComboBoxItem();
11: item.Name = voice.DisplayName;
12: item.Tag = voice;
13: item.Content = voice.DisplayName;
14: this.VoiceComboBox.Items.Add(item);
15:
16: if (currentVoice.Id == voice.Id)
17: {
18: item.IsSelected = true;
19: this.VoiceComboBox.SelectedItem = item;
20: }
21: }
When we now click on the Button, we need to indicate the text we want to read out. Since this is a call that can take some time, it’s an async process. This returns a SynthesisStream instance. If the latter isn’t null, we use this stream as the source for the MediaElement. This MediaElement has its AutoPlay property set to true so it will start playing automatically.
1: try
2: {
3: synthesisStream = await this.speechSynthesizer.SynthesizeTextToStreamAsync(MainTextBox.Text);
4: }
5: catch (Exception ex)
6: {
7: synthesisStream = null;
8: }
9:
10: if (synthesisStream != null)
11: {
12: MainMediaElement.AutoPlay = true;
13: MainMediaElement.SetSource(synthesisStream, synthesisStream.ContentType);
14: MainMediaElement.Play();
15: }
16: else
17: {
18: MessageDialog dialog = new MessageDialog("unable to synthesize text");
19: await dialog.ShowAsync();
20: }
The Contact Manager classes
In Windows Phone, it has been possible for some time to allow apps to manage the contacts of the user as well as manage the calendar. This feature was so far missing in Windows 8, therefore, it was hard to create apps that interact with the calendar or provide address book interactions.
With Windows 8.1, an API is available to do this, namely the contact manager API and the appointments API. Let’s start by looking at the first one.
In the Windows.ApplicationModel.Contacts namespace, we find all kind of classes that allow us to interact with the contact we have in the people app. The most important classes here are the Contact and the ContactManager. The Contact class represents a contact; we have all kinds of related classes such as ContactAddress, ContactEmail and ContactJobInfo. All of these allow us to represent parts of the “contact card” of a contact. That contact card is an interesting aspect of the ContactManager class. The latter allows us to enter information and we can search for a contact. Once found, we get back a contact card, shown below.
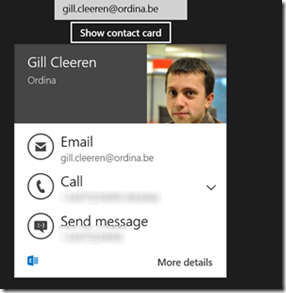
Let’s see in code how we can do this.
First, we get the contact email. For this, we use the ContactEmail class. We assign this to the Contact we also create.
1: Contact contact = new Contact();
2: ContactEmail contactEmail = new ContactEmail();
3: contactEmail.Address = EmailAddressTextBox.Text;
4: contact.Emails.Add(contactEmail);
Next, we use the ContactManager class to retrieve all the information about the contact we find on the system.
1: FrameworkElement fe = sender as FrameworkElement;
2:
3: // Get the selection rect of the button pressed to show contact card.
4: Windows.UI.Xaml.Media.GeneralTransform buttonTransform = fe.TransformToVisual(null);
5: Point point = buttonTransform.TransformPoint(new Point());
6: var rect = new Rect(point, new Size(fe.ActualWidth, fe.ActualHeight));
7:
8: ContactManager.ShowContactCard(contact, rect, Windows.UI.Popups.Placement.Default);
Other noteworthy changes
We’ve now already covered a number of interesting classes. But there are other interesting changes.
Geofencing support
Geofencing is becoming more and more used. Perhaps first: what’s a geofence? As the word implies, it’s an imaginary fence around a certain point. When the device enters the geofence, we can choose to run code. This enables again some cool scenarios. Imagine you’re building an app for Starbucks. In the app, you can create geofences around all Starbucks’s. When the device enters a geofence, the app will be notified about this. Or you can create a geofence around yourself and when you’re in the vicinity of one of your friends, the app can be notified as well.
When the app is notified about this, we can run code. This is possible with a new type of trigger, the LocationTrigger. With this trigger, we can trigger a background task that then will for example show a toast notification about the fact you’re close to a Starbucks to grab a cup of coffee!
Changes to the BackgroundTransfer API
When uploading or downloading files, we can use the BackgroundTransfer API. Using this API, it’s possible to register transfers with a background process that won’t be suspended when the main app goes into the suspended mode.
Some improvements were made to this API. It’s now possible to use a BackgroundTransferGroup. This class lets us group transfers and assign them a priority. Also, we can now notify the user about the fact a transfer is finished using a tile or a toast update. The latter is an interesting feature, since previously, it wasn’t possible to let the user know that a transfer was ready.
POS support
The last interesting aspect we’ll talk about is better devices support and POS (Point-Of-Service) support. Windows 8.1 adds much better support for hardware, including USB, Bluetooth, HID (Human Interface Device) and even 3D printing.
POS support is probably the most interesting one. It enables us to build cash register systems since we can now talk to magnetic swipe readers and barcode scanners.
Summary
In this third part, we’ve discussed some more WinRT classes that enable some cool scenarios. In the next article, we’ll discuss UX related topics, including the new size options. See you there!
About the author
Gill Cleeren is Microsoft Regional Director, Silverlight MVP, Pluralsight trainer and Telerik MVP. He lives in Belgium where he works as .NET architect at Ordina. Gill has given many sessions, webcasts and trainings on new as well as existing technologies, such as Silverlight, ASP.NET and WPF at conferences including TechEd, TechDays, DevDays, NDC Oslo, SQL Server Saturday Switzerland, Silverlight Roadshow in Sweden, Telerik RoadShow UK… Gill has written 2 books: “Silverlight 4 Data and Services Cookbook” and Silverlight 5 Data and Services Cookbook and is author of many articles for magazines and websites. You can find his blog at www.snowball.be. Twitter: @gillcleeren