This article is compatible with the latest version of Silverlight.
This is the first of series of articles about the DataGrid control. In it I'll introduce you to the basics of the controls how to add data to it and how to define its columns. With every next article I'm going to add something new to this example and keep extending it with cool things, so at the end there will be a lot of information about the DataGrid, some Tips and Tricks and a lot of source code and demos. Now let's start with the basics and don't be impatient - soon the things will get very interesting!
Adding a DataGrid control
From the ToolBox we drag and drop a Datagrid control into the Xaml. The first thing that will make an impression to you is the tag and the "my" prefix:
<my:DataGrid x:Name="Foods"></my:DataGrid>
That's because the DataGrid is found in the System.Windows.Controls namespace. If you take a look at your UserControl tag you'll see that a declaration for this namespace was generated in it:
<UserControl x:Class="DataGrid.Page"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:my="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data"
Width="400" Height="300">
Configuring the columns
For now we use the ability of the DataGrid to auto generate columns for each property of the source object. We do that by setting the AutoGenerateColumns property to true. Later we'll see how to manually define the columns:
<my:DataGrid x:Name="Food" AutoGenerateColumns="True"></my:DataGrid>
Adding some data
What is the DataGrid without a data in it? Nothing, so let's add some data. For my examples I will use the same information Emil has used in his article about Creating a simple Pivot table using LINQ and RadTreeView for Silverlight, namely the nutrition that are contained in the pizza. Let's start with creating the Xml file:
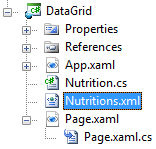
and it contains:
<?xml version="1.0" encoding="utf-8" ?>
<Nutritions>
<Nutrition Group="Carbohydrates" Name="Total carbohydrates" Quantity="27.3"></Nutrition>
<Nutrition Group="Carbohydrates" Name="Total disaccharides" Quantity="5.7"></Nutrition>
<Nutrition Group="Carbohydrates" Name="Total polysaccharides" Quantity="21.6"></Nutrition>
<Nutrition Group="Minerals" Name="Calcium" Quantity="147"></Nutrition>
<Nutrition Group="Minerals" Name="Phosphorus " Quantity="150"></Nutrition>
<Nutrition Group="Minerals" Name="Potassium " Quantity="201"></Nutrition>
<Nutrition Group="Minerals" Name="Copper " Quantity="0.13"></Nutrition>
<Nutrition Group="Minerals" Name="Magnesium " Quantity="19"></Nutrition>
<Nutrition Group="Minerals" Name="Sodium " Quantity="582"></Nutrition>
<Nutrition Group="Minerals" Name="Selenium " Quantity="4"></Nutrition>
<Nutrition Group="Minerals" Name="Total iron " Quantity="0.7"></Nutrition>
<Nutrition Group="Minerals" Name="Zinc" Quantity="1.07"></Nutrition>
<Nutrition Group="Vitamins" Name="Beta-carotene " Quantity="173.8"></Nutrition>
<Nutrition Group="Vitamins" Name="Nicotinic " Quantity="1.5"></Nutrition>
<Nutrition Group="Vitamins" Name="Total vitamin B6 " Quantity="0.127"></Nutrition>
<Nutrition Group="Vitamins" Name="Total vitamin D" Quantity="0.3"></Nutrition>
<Nutrition Group="Vitamins" Name="Total vitamin E " Quantity="2.1"></Nutrition>
<Nutrition Group="Vitamins" Name="Vitamin B1 " Quantity="0.1"></Nutrition>
<Nutrition Group="Vitamins" Name="Vitamin B12 " Quantity="0.59"></Nutrition>
<Nutrition Group="Vitamins" Name="Vitamin B2" Quantity="0.16"></Nutrition>
<Nutrition Group="Vitamins" Name="Vitamin C" Quantity="10"></Nutrition>
</Nutritions>
We will use LINQ to read the Xml file and get the information about each nutrition. If you want to know about how to use LINQ for reading or writing Xml documents, take a look at this article. I also create a class called Nutrition which has three properties - Group, Name, Quantity. Now what we need is to extract a list of Nutritions from the Xml and set it as source of our DataGrid. The ItemsSource property is used for that purpose. Here is the code:
private void LoadData()
{
XDocument nutritionsDoc = XDocument.Load( "Nutritions.xml" );
List<Nutrition> data = ( from nutrition in nutritionsDoc.Descendants( "Nutrition" )
select new Nutrition
{
Group = nutrition.Attribute( "Group" ).Value,
Name = nutrition.Attribute( "Name" ).Value,
Quantity = nutrition.Attribute( "Quantity" ).Value
} ).ToList();
Foods.ItemsSource = data;
}
And here is the result:
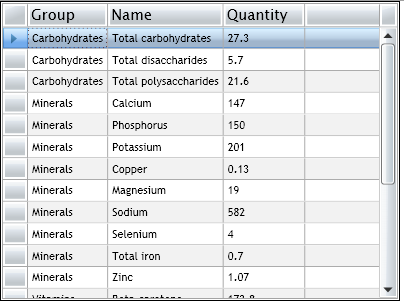
Manually defining the columns
When we know exactly what properties the object has or when we want not every property to be shown as column, we can manually define the columns and the bindings. In this case we need Text columns, but in the next article we'll see the other types of columns.
Xaml
<my:DataGrid x:Name="Foods">
<my:DataGrid.Columns>
<my:DataGridTextColumn Binding="{Binding Group}" Header="Group"></my:DataGridTextColumn>
<my:DataGridTextColumn Binding="{Binding Name}" Header="Name"></my:DataGridTextColumn>
<my:DataGridTextColumn Binding="{Binding Quantity}" Header="Quantity"></my:DataGridTextColumn>
</my:DataGrid.Columns>
</my:DataGrid>
C#
DataGridTextColumn textColumn1 = new DataGridTextColumn();
textColumn1.Header = "Group";
textColumn1.Binding = new Binding( "Group" );
Foods.Columns.Add( textColumn1 );
DataGridTextColumn textColumn2 = new DataGridTextColumn();
textColumn2.Header = "Name";
textColumn2.Binding = new Binding( "Name" );
Foods.Columns.Add( textColumn2 );
DataGridTextColumn textColumn3 = new DataGridTextColumn();
textColumn3.Header = "Quantity";
textColumn3.Binding = new Binding( "Quantity" );
Foods.Columns.Add( textColumn3 );
And finally some Silverlight:
As you can see by default we have selection and sorting by columns functionality in the grid, but these are things that we'll take a much closer look soon.
Summary
Now we know how to create a DataGrid control, how to bind some data to it and how to define it's columns. The next time we're going to extend this example by adding some styles and templates and other cool things. So stay tuned!