This is Day # 7 in the Windows 8 development article series on common tips & tricks towards real-world Windows 8 Store apps. Now that we’ve got our Windows 8 App working, time to provide the always-on & always-connected user experience – leveraging services the right way. Over the last several weeks, you saw 8 articles talk about some must-do things for Windows 8 app developers. Simple & to the point, with some code examples on XAML/C# stack. Here’s the indexed list for the series:
Day 1: Know the ecosystem; Start
Day 2: Layout, Navigation & Visual States
Day 3: Semantic Zoom
Day 4: Controls & Styling
Day 5: Search, Share & Settings Contracts
Day 6: Data Persistence & Application Life-Cycle Management
Day 7: Use of OData or Web Services
Day 8: Live Services integration
Day 7: Use of OData or Web Services
Connected & Alive – one of key guiding principles of Modern UI Design Language that contributes towards the user experience provided by Windows 8 style Apps. How can the Start screen be lit up with customized content and innovative Tiles? How can Apps not be running, but yet appearing to have fresh data/content? How can one have Apps feel connected, even without network connectivity? Well, through the use of services that reside outside the Windows 8 App we are making. It is this outside partner to our App that often does some heavy lifting in processing logic to make the App feel alive & connected. It is important to understand how to leverage services to manage data and push back content to our mobile Apps. Let’s explore our options on XAML/C# stack ..
Connectivity to Services
Few Windows 8 Apps would come alive without access to some network data. Many a times, we may be pulling down resources from web URLs or RESTful services. What’s the easiest way? Why off course, the HTTPClient class in the System.Net.Http namespace. It can be used to send basic HTTP requests in the form of GET, PUT, POST & DELETE and receive HTTP responses back, all asynchronous in Windows 8.
For example, here’s some sample code to pull down a SilverlightShow article:
1: HttpClient httpClient = new HttpClient();
2: HttpResponseMessage response = await httpClient.GetAsync("http://www.silverlightshow.net/items/Windows-8-Apps-The-8-Must-Know-Tricks-Day-6.aspx");
3: string articleAsHTMLString = await response.Content.ReadAsStringAsync();
As you can see, this is pretty simple. What you do with the response and how you bind it to your UI is up to what your application needs. However, one thing to remember is that before you make any network calls from Windows 8 Store Apps, it must be declared explicitly as a Capability that the application needs in the corresponding .AppManifest file, as shown below.
Now, the above is only the simplest example of accessing a network resource from Windows 8 Apps; there are off course, other forms of connectivity, like pulling down RSS Feeds using SyndicationFeed classes, secure connections over HTTPS, two way client-server communication over WebSockets protocol or low level connections over TCP/UDP. For more information, a great starting point would be http://msdn.microsoft.com/en-us/library/windows/apps/xaml/br229573.aspx.
Windows 8 Apps can also talk more intimately with hosted Web Services, if we add references to the Service in our project. With a service reference, our code could have access to service data types & have tight coupling with object structures on the service side, to invoke methods for connectivity, obviously in an asynchronous way. While this is true for most WCF or RESTful services, we shall see examples of this for an OData service, as below.
OData
The Open Data Protocol (OData), as we know, is an HTTP based protocol for querying and updating data through a service layer that is easily configurable; more details @ http://www.odata.org/. Handing out data in ATOM/JSon formats, OData extends itself to use from multitude of platforms, and yes natively from Windows 8 Store Apps. While we can absolutely hand-code the HTTP requests, .NET wrappers for the XAML/C# world make it really easy to query/update data back on a service. In fact, OData may be considered to be one of the easiest ways to support Database CRUD (Create, Read, Update. Delete) from a Windows 8 style App.
One of the advantages of having easy access to a Service that handles data CRUD is the seamless connectivity it offers for Windows 8 Style Apps. We can lazy load data opportunistically and the user can have latest data locally, make edits & push it back to the OData service for syncing with the server. This particularly facilitates the scenario of Technicians or other types of users going out to areas of no connectivity with Windows 8 tablets and yet being able to sync data easily (automatically or by trigger) when network is available. Local data, with changes, can be persisted in serialized objects Isolated Storage or through the use of SQLLite for truly relational data. And classes like DataServiceCollection and LINQ techniques make handling the data a breeze – pull down filtered data, bind locally to UI and when done with changes, easily update back up to the OData service.
Here are few of the steps you need to integrate OData service usage from a Windows 8 Store App:
- First host the OData service or use one that is already available for your cause. Just about any relational data can be exposed as an OData feed with little orchestration, but allowing complete CRUD operations on data, over proper authentication. For more info on how to create an OData service, start with Michael Crump’s brilliantly simple article series @ http://www.silverlightshow.net/items/Producing-and-Consuming-OData-in-a-Silverlight-and-Windows-Phone-7-application.aspx.
- Check the OData service endpoint in a browser to make sure that all the HTTP collection URLs are returning data as expected.
- Next, download the WCF Data Services Tools for Windows Store Apps from http://www.microsoft.com/en-us/download/details.aspx?id=30714. This enables all the plumbing needed to easily add a Service Reference to the OData service endpoint.
- Fire up your Windows 8 Store App project in Visual Studio and simply add a Service Reference to the OData service.
Using the quintessential Netflix OData service feed found at http://odata.netflix.com/catalog/ as an example, our Service Reference addition is trivial, and it immediately gives our project access to the data types exposed in the OData service:
Fetching data down from an OData service is trivial, with our tooling making asynchronous HTTP GET calls behind the scenes:
1: using System.Data.Services.Client;
2:
3: // Reference to Context & Object Collection from OData Service.
4: DataServiceContext oDataContext;
5: DataServiceCollection<SomeCustomObjectType> objectListFromService;
6:
7: // Instantiate the context.
8: oDataContext = new DataServiceContext(new Uri("OData EndPoint"));
9: objectListFromService = new DataServiceCollection<SomeCustomObjectType>(oDataContext);
10:
11: // Reach the entities.
12: Uri uriQuery = new Uri("/SomeCustomHTTPEndpoint", UriKind.Relative);
13:
14: // Asynchronously load the fresh data from Service.
15: objectListFromService.LoadAsync(uriQuery);
16:
17: objectListFromService.LoadCompleted += (sender, args) =>
18: {
19: if (args.Error != null)
20: {
21: // Do some error handling.
22: }
23: else
24: {
25: // Success.
26: // Bind to UI.
27: }
28: };
Once fetched, the data objects could be serialized and saved locally. If there are updates to made, we could simply locate the object which needs to change, update it’s properties, mark it dirty & send HTTP POST requests back to the OData service to update records in the backend. The beauty is, this can all be done without writing any low-level HTTP stuff, but rather simple .NET code, as show below:
1: // Find the object to update from local list.
2: SomeCustomObjectType serviceObj = objectListFromService.First(T => [some predicate expression]);
3:
4: serviceObj.Prop1 = ...
5: serviceObj.Prop2 = ...
6:
7: // Mark the object dirty & ready for update to DB.
8: oDataContext.UpdateObject(serviceObj);
9:
10: // Commit all at once.
11: oDataContext.BeginSaveChanges(new AsyncCallback(SaveDoneCB), null);
12:
13: private void SaveDoneCB(IAsyncResult asynchronousResult)
14: {
15: // On a different thread here!
16: if (asynchronousResult.IsCompleted)
17: {
18: // Indicate completion.
19: // Bubble event up to any listeners or update UI.
20: }
21: }
Push Notifications
Now, the other huge usage of Services for Windows 8 Store Apps is in supporting Push Notifications. This truly contributes to the connected and alive user experience in Windows 8 Apps, by feeding Live Tiles on the Start screen or Toast notifications. The basics of Push Notification on Windows 8 is captured by the following:
Few steps to get this working are:
- Windows 8 App client requests an unique Channel URI through the PushNotificationChannelManager from WNS.
- Once received, the App sends up the Channel URI to some hosted Web Service, preferably over a secure connection. This is where the service integration comes into play. This other companion service to the App could be hosted anywhere and may be a WCF/RESTful/regular web service. The client app may have a reference to this service & needs to invoke methods on the service to send up the channel URI. Or in case of a RESTful service, a simple HTTP POST would be enough to send up the channel URI.
- Next, our service will authenticate itself with Windows Push Notification Service (WNS) through some handshaking of tokens and send down push notification packets at the given channel URIs through a secured HTTP POST.
- WNS turns around and delivers the push packets down to each Windows 8 devices.
- Before our cloud web service can utilize WNS to send Push Notifications, the App needs to be registered through the Windows Dashboard to provide authenticating credentials. These take the form of a Package Identifier & a security key, which is sent up to the cloud service. The cloud service uses the credentials to handshake with WNS through OAuth and receives an access token, which is utilized across every WNS POST request.
- For more detailed information, please see http://msdn.microsoft.com/en-us/library/windows/apps/xaml/hh913756.aspx.
Tiles & Toasts
Although we primarily talked service integrations here, it is worth mentioning what the Push Notification services fuel. Live Tiles on the Start screen are live representations of each App; they are the gateway to the App & need to impress and then continue to invite the user back into the application. Tiles are available in two sizes – square and wide; and can be any combination of text, images and notifications called badges. Tiles are based on a non-extensible set of predefined XML templates, so that the OS may know how to process them and can truly be used to push through App branding. Also, tiles can cycle through the last 5 received push notifications for an enticing peak animation, thus inviting the user back. And just like in Windows Phone, there is support for Secondary Tiles, which can pin specific sections of the App to the Start screen & take the user back to a particular sub-experience when tapped. More information on Tiles available @ http://msdn.microsoft.com/en-us/library/windows/apps/xaml/hh779724.aspx.
A Toast, on the other hand, is a transient notification that pops up on the top right edge of the Windows 8 screen, irrespective of where the user is at. Toasts are meant to provide relevant, time-sensitive information to users & allow the user to quickly jump into related content in the App which triggered the Toast notification. Since this can be intrusive and only used for high-interest content, a capability declaration is needed in the App Manifest file and the user has the option of turning off notifications. A Toast notification is made up of texts/images and just like Tiles, supported through pre-defined templates. More detailed information about Toasts is over @ http://msdn.microsoft.com/en-us/library/windows/apps/xaml/hh779727.aspx.
An assortment of Tile & Toast templates is shown below:
Image courtesy – Microsoft.
Conclusion
That’s it for today. The crux of this article was to talk about Service integration from Windows 8 Store Apps. In particular,we saw how OData service could fuel CRUD operations from our Apps and then how to have Push Notifications supported through our cloud service, thus feeding various Tiles & Toasts.
See you next time as we dive into Live Services integration in Windows 8 Apps. Thanks for reading!
About Author
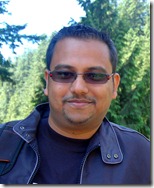
Samidip Basu (@samidip) is a technologist, gadget-lover and MSFT Mobility Solutions Lead for Sogeti USA working out of Columbus OH. With a strong developer background in Microsoft technology stack, he now spends much of his time evangelizing Windows Phone/Windows 8 platforms & cloud-supported mobile solutions in general. He passionately helps run The Windows Developer User Group (http://thewindowsdeveloperusergroup.com/), labors in M3 Conf (http://m3conf.com/) organization and can be found with at-least a couple of hobbyist projects at any time. His spare times call for travel and culinary adventures with the wife. Find out more athttp://samidipbasu.com.